38 releases
0.11.4 | Jul 17, 2024 |
---|---|
0.11.2 | Feb 27, 2024 |
0.9.1 | Dec 29, 2023 |
0.9.0 | Sep 8, 2023 |
0.1.0 | Jul 5, 2020 |
#3 in Rendering engine
1,896 downloads per month
5MB
16K
SLoC
Screen 13
Screen 13 is an easy-to-use Vulkan rendering engine in the spirit of QBasic.
[dependencies]
screen-13 = "0.11"
Overview
Screen 13 provides a high performance Vulkan driver using smart pointers. The driver may be created manually for headless rendering or automatically using the built-in event loop abstraction:
use screen_13::prelude::*;
fn main() -> Result<(), DisplayError> {
EventLoop::new().build()?.run(|frame| {
// It's time to do some graphics! 😲
})
}
Usage
Screen 13 provides a fully-generic render graph structure for simple and statically
typed access to all the resources used while rendering. The RenderGraph
structure allows Vulkan
smart pointer resources to be bound as "nodes" which may be used anywhere in a graph. The graph
itself is not tied to swapchain access and may be used to execute general command streams.
Features of the render graph:
- Compute, graphic, and ray-trace pipelines
- Automatic Vulkan management (render passes, subpasses, descriptors, pools, etc.)
- Automatic render pass scheduling, re-ordering, merging, with resource aliasing
- Interoperable with existing Vulkan code
- Optional shader hot-reload from disk
render_graph
.begin_pass("Fancy new algorithm for shading a moving character who is actively on fire")
.bind_pipeline(&gfx_pipeline)
.read_descriptor(0, some_image)
.read_descriptor(1, another_image)
.read_descriptor(3, some_buf)
.clear_color(0, swapchain_image)
.store_color(0, swapchain_image)
.record_subpass(move |subpass| {
subpass.push_constants(some_u8_slice);
subpass.draw(6, 1, 0, 0);
});
Debug Logging
This crate uses log
for low-overhead logging.
To enable logging, set the RUST_LOG
environment variable to trace
, debug
, info
, warn
or
error
and initialize the logging provider of your choice. Examples use
pretty_env_logger
.
You may also filter messages, for example:
RUST_LOG=screen_13::driver=trace,screen_13=warn cargo run --example ray_trace
TRACE screen_13::driver::instance > created a Vulkan instance
DEBUG screen_13::driver::physical_device > physical device: NVIDIA GeForce RTX 3090
DEBUG screen_13::driver::physical_device > extension "VK_KHR_16bit_storage" v1
DEBUG screen_13::driver::physical_device > extension "VK_KHR_8bit_storage" v1
DEBUG screen_13::driver::physical_device > extension "VK_KHR_acceleration_structure" v13
...
Performance Profiling
This crates uses profiling
and supports multiple profiling
providers. When not in use profiling has zero cost.
To enable profiling, compile with one of the profile-with-*
features enabled and initialize the
profiling provider of your choice.
Example code uses puffin:
cargo run --features profile-with-puffin --release --example vsm_omni
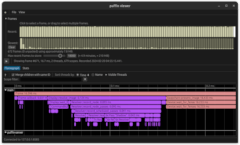
Quick Start
Included are some examples you might find helpful:
hello_world.rs
— Displays a window on the screen. Please start here.triangle.rs
— Shaders and full setup of index/vertex buffers; < 100 LOC.shader-toy/
— Recreation of a two-pass shader toy using the original shader code.
See the example code, documentation, or helpful getting started guide for more information.
NOTE: Required development packages and libraries are listed in the getting started guide. All new users should read and understand the guide.
History
As a child I was given access to a computer that had GW-Basic; and later one with QBasic. All of my favorite programs started with:
CLS
SCREEN 13
These commands cleared the screen of text and setup a 320x200 256-color paletized video mode. There were other video modes available, but none of them had the 'magic' of 256 colors.
Additional commands QBasic offered, such as DRAW
, allowed you to build simple games quickly
because you didn't have to grok the entirety of compiling and linking. I think we should have
options like this today, and so I started this project to allow future developers to have the
ability to get things done quickly while using modern tools.
Inspirations
Screen 13 was built from the learnings and lessons shared by others throughout our community. In particular, here are some of the repositories I found useful:
Dependencies
~10–48MB
~748K SLoC