17 releases
0.3.7 | Jul 19, 2024 |
---|---|
0.3.1 | Mar 11, 2024 |
0.2.2 | Dec 28, 2023 |
0.2.1 | Sep 17, 2023 |
0.1.7 | May 18, 2023 |
#2488 in Database interfaces
330 downloads per month
Used in 2 crates
(via welds)
51KB
1.5K
SLoC
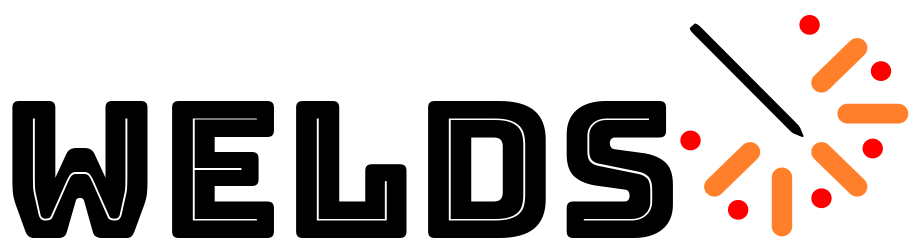
An async ORM written in rust using sqlx and/or Tiberius.
Welds
Welds is an async ORM written in rust using sqlx and/or Tiberius.
Features
- Async for all.
- Support for multiple SQL databases (Mssql, MySql, Postgres, Sqlite)
- Written for ease of development. Features aren't hidden behind traits. Code should be simple to write, and simple to read.
- Low level connection always available when you need to drop down to raw SQL.
Under the hood welds uses:
- sqlx for Postgres, MySql, and Sqlite.
- Tiberius for MSSQL
Example Setup
#[derive(Debug, WeldsModel)]
#[welds(schema= "inventory", table = "products")]
#[welds(BelongsTo(seller, super::people::People, "seller_id"))]
pub struct Product {
#[welds(rename = "product_id")]
#[welds(primary_key)]
pub id: i32,
pub name: String,
pub seller_id: Option<i32>,
pub description: Option<String>,
pub price: Option<f32>,
}
Example Usage
Basic Select
let url = "postgres://postgres:password@localhost:5432";
let client = welds::connections::postgres::connect(url).await.unwrap();
let products = Product::where_col(|p| p.price.equal(3.50)).run(&client).await?;
Basic Filter Across tables
let client = welds::connections::mssql::connect(url).await.unwrap();
let sellers = Product::where_col(|product| product.price.equal(3.50))
.map_query(|product| product.seller )
.where_col(|seller| seller.name.ilike("%Nessie%") )
.run(&client).await?;
Create And Update
let client = welds::connections::sqlite::connect(url).await.unwrap();
let mut cookies = Product::new();
cookies.name = "cookies".to_owned();
// Creates the product cookie
cookies.save.await(&client)?;
cookies.description = "Yum".to_owned();
// Updates the Cookies
cookies.save.await(&client)?;
Other Examples
- Basic CRUD
- Mapping Queries / Joining
- Bulk (Create/Update/Delete)
- Select Only Specific Columns
- Checking DB schema matches compiled structs
For more good examples check out the examples repo.
Dependencies
~1.5MB
~37K SLoC