29 releases
0.4.10 | Jan 1, 2025 |
---|---|
0.4.8 | Nov 22, 2024 |
0.3.7 | Jul 19, 2024 |
0.3.1 | Mar 11, 2024 |
0.1.7 | May 18, 2023 |
#85 in Database interfaces
789 downloads per month
Used in 2 crates
360KB
9K
SLoC
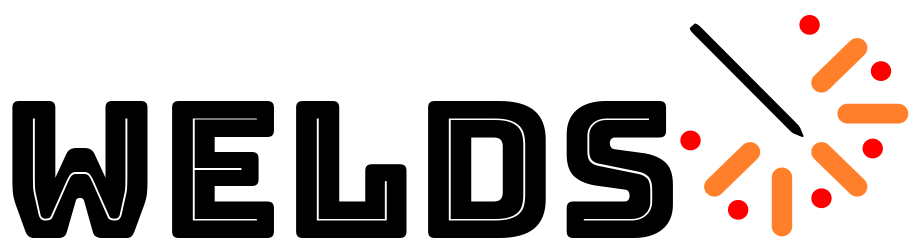
An async ORM written in rust using sqlx and/or Tiberius.
Welds
Welds is an async ORM written in rust using sqlx and/or Tiberius.
Features
- Async for all.
- Support for multiple SQL databases (Mssql, MySql, Postgres, Sqlite)
- Written for ease of development. Features aren't hidden behind traits. Code should be simple to write, and simple to read.
- Low level connection always available when you need to drop down to raw SQL.
Under the hood welds uses:
- sqlx for Postgres, MySql, and Sqlite.
- Tiberius for MSSQL
Compatibility:
- the
0.4.*
line of welds is compiled with sqlx 0.8 - the
0.3.*
line of welds is compiled with sqlx 0.7
Example Setup
#[derive(Debug, WeldsModel)]
#[welds(schema= "inventory", table = "products")]
#[welds(BelongsTo(seller, super::people::People, "seller_id"))]
pub struct Product {
#[welds(rename = "product_id")]
#[welds(primary_key)]
pub id: i32,
pub name: String,
pub seller_id: Option<i32>,
pub description: Option<String>,
pub price: Option<f32>,
}
Example Usage
Basic Select
let url = "postgres://postgres:password@localhost:5432";
let client = welds::connections::postgres::connect(url).await.unwrap();
let products = Product::where_col(|p| p.price.equal(3.50)).run(&client).await?;
Basic Filter Across tables
let client = welds::connections::mssql::connect(url).await.unwrap();
let sellers = Product::where_col(|product| product.price.equal(3.50))
.map_query(|product| product.seller )
.where_col(|seller| seller.name.ilike("%Nessie%") )
.run(&client).await?;
Create And Update
let client = welds::connections::sqlite::connect(url).await.unwrap();
let mut cookies = Product::new();
cookies.name = "cookies".to_owned();
// Creates the product cookie
cookies.save.await(&client)?;
cookies.description = "Yum".to_owned();
// Updates the Cookies
cookies.save.await(&client)?;
Types from external crates
Both Tiberius
and sqlx
support types from external crates such at chrono
and serde_json
. These types need to be enabled in the underlying crate to use.
In order to use types that are external the appropriate feature needs to be enabled in these underlying frameworks.
We have chosen to leave this up to you as the developer so you have full control over your underlying SQLX/Tiberius setup.
In order to get these types to work you will need to:
- Add the external crate
cargo add chrono
- Enable the feature in the underlying SQL framework.
cargo add sqlx --features=chrono
- (Tiberius only) enable the corresponding feature for welds-connections feature
cargo add welds-connections --features=mssql,mssql-chrono
welds-connections features needed for mssql (tiberius):
- mssql-chrono
- mssql-time
- mssql-rust_decimal
- mssql-bigdecimal
Other Examples
- Basic CRUD
- Mapping Queries / Joining
- Bulk (Create/Update/Delete)
- Select Only Specific Columns
- Hooks, Callback when models (Save/Update/Delete)
- Migrations
- Checking DB schema matches compiled structs
For more good examples check out the examples repo.
Dependencies
~0.4–17MB
~248K SLoC