1 unstable release
0.1.0 | May 28, 2020 |
---|
#6 in #fonts
24 downloads per month
Used in mints
26KB
630 lines
Terminal Fonts
Big fonts for terminal display. Each character is a block formed by many dots.
The font data came from Arcade, font copy rights belongs to the original author.
Usage
Generate block string directly
use terminal_fonts::{to_block_string};
fn main() {
println!("{}", to_block_string("05:30:12 AM"))
}
███ ██████ ██████ ███ ██ █████ ███ ██ ██
█ ██ ██ ██ ██ █ ██ ██ ███ ██ ██ ██ ██ ███ ███
██ ██ ██████ ██ ██ ██ ██ ██ ██ ███ ██ ██ ███████
██ ██ ██ ████ ██ ██ ██ ████ ██ ██ ███████
██ ██ ██ ██ ██ ██ ██ ████ ███████ ██ █ ██
██ █ ██ ██ ██ ██ ██ ██ █ ██ ██ ███ ██ ██ ██ ██
███ █████ ██ █████ ███ ██ ██████ ███████ ██ ██ ██ ██
Generate blocks and manipulate them
use terminal_fonts::{map_block, to_block, concat_blocks, to_string};
fn red(v: &str) -> String {
format!("{}{}{}", "\u{001b}[31m", v, "\u{001b}[0m")
}
fn yellow(v: &str) -> String {
format!("{}{}{}", "\u{001b}[33m", v, "\u{001b}[0m")
}
fn blue(v: &str) -> String {
format!("{}{}{}", "\u{001b}[34m", v, "\u{001b}[0m")
}
fn main() {
let hour_block = map_block(&to_block("05"), red);
let minute_block = map_block(&to_block("30"), yellow);
let second_block = map_block(&to_block("12"), blue);
let sep_block = to_block(":");
let result = to_string(&concat_blocks(&vec![
&hour_block,
&sep_block,
&minute_block,
&sep_block,
&second_block,
]));
println!("{}", result)
}
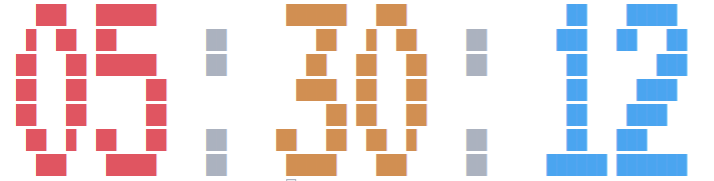
Dependencies
~10KB