17 releases
0.5.4 | Dec 15, 2020 |
---|---|
0.5.3 | Nov 21, 2020 |
0.5.2 | Jan 25, 2020 |
0.4.1 | Jan 19, 2020 |
0.1.3 | Jan 10, 2020 |
#798 in Graphics APIs
1.5MB
2K
SLoC
Overview
A library that lets you draw various simple 2d geometry primitives and sprites fast using Vertex buffer objects with a safe api. Uses the builder pattern for a convinient api. The main design goal is to be able to draw thousands of shapes efficiently. Uses glutin and opengl es 3.0.
Screenshot
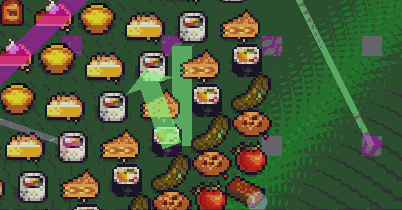
Example
See the github example, or see the crate documentation.
Demo
Demo sprites are provided courtesy of:
- Henry Software - https://henrysoftware.itch.io/pixel-food
- rvros - https://rvros.itch.io/animated-pixel-hero
- savvycow - https://savvycow.itch.io/loudypixelsky
lib.rs
:
Overview
A library that lets you draw various simple 2d geometry primitives and sprites fast using vertex buffer objects with a safe api. Uses the builder pattern for a convinient api. The main design goal is to be able to draw thousands of shapes efficiently. Uses glutin and opengl es 3.0.
Pipeline
The egaku2d drawing pipeline works as follows:
-
- Pick a drawing type (a particular shape or a sprite) and set mandatory values for the particular shape or sprite.
-
- Build up a large group of verticies by calling
add()
- 2.1 Optionally save off verticies to a static vbo on the gpu for fast drawing at a later time by calling
save()
.
- Build up a large group of verticies by calling
-
- Send the vertex data to the gpu and set mandatory shader uniform values bt calling
send_and_uniforms()
- 3.1 Set optional uniform values e.g.
with_color()
.
- Send the vertex data to the gpu and set mandatory shader uniform values bt calling
-
- Draw the verticies by calling
draw()
- Draw the verticies by calling
Additionally, there is a way to draw the vertices we saved off to the gpu.
To do that, instead of steps 1 and 2, we use the saved off verticies,
and then set the uniform values by valling uniforms()
and then draw by calling draw()
.
Using this pipeline, the user can efficiently draw thousands of circles, for example, with the caveat that they all will be the same radius and color/transparency values. This api does not allow the user to efficiently draw thousands of circles where each circle has a different color or radius. This was a design decision to make each vertex as lightweight as possible (just a x and y position), making it more efficient to set and send to the gpu.
Key Design Goals
The main goal was to make a very performat simple 2d graphics library. There is special focus on reducing traffic between the cpu and the gpu by using compact vertices, point sprites, and by allowing the user to save vertex data to the gpu on their own.
Providing a safe api is also a goal. All draw functions require a mutable version to the canvas, ensuring they happen sequentially. The user is prevented from making multiple instances of the system using an atomic counter. The system also does not implement Send so that the drop calls from vertex buffers going out of scope happen sequentially as well. If the user were to call opengl functions on their own, then some safety guarentees might be lost. However, if the user does not, this api should be completely safe.
Writing fast shader programs is a seconady goal. This is a 2d drawing library even though most of the hardware out there
is made to handle 3d. This means that the gpu is most likely under-utilized with this library.
Because of this, it was decided there is little point to make a non-rotatable sprite shader to save
on gpu time, for example. Especially since the vertex layout is the same size (with 32bit alignment) ([f32;2],i16,i16
vs [f32;2],i16
),
so there are no gains from having to send less data to the gpu.
Using Shapes
The user can draw the following:
Shape | Representation | Opengl Primitive Type |
---|---|---|
Circles | (point,radius) |
POINTS |
Axis Aligned Rectangles | (startx,endx,starty,endy) |
TRIANGLES |
Axis Aligned Squares | (point,radius) |
POINTS |
Lines | (point,point,thickness) |
TRIANGLES |
Arrows | (point_start,point_end,thickness) |
TRIANGLES |
Using Sprites
This crate also allows the user to draw sprites. You can upload a tileset texture to the gpu and then draw thousands of sprites using a similar api to the shape drawing api. The sprites are point sprites drawn using the opengl POINTS primitive in order to cut down on the data that needs to be sent to the gpu.
Each sprite vertex is composed of the following:
- position:
[f32;2]
- index:
u16
- the user can index up to 256*256 different sprites in a tile set. - rotation:
u16
- this gets normalized to a float internally. The user passes a f32 float in radians.
So each sprite vertex is compact at 4*3=12 bytes.
Each texture object has functions to create this index from a x and y coordinate. On the gpu, the index will be split into a x and y coordinate. If the index is larger than texture.dim.x*texture.dim.y then it will be modded so that it can be mapped to a tile set. Therefore it is impossible for the index to have a 'invalid' value. But obviously, the user should be picking an index that maps to a valid tile in the tile set to begin with.
The rotation is normalized to a float on the gpu. The fact that the tile index has size u16, means you can have a texture with a mamimum of 256x256 tiles. The user simply passes a f32 through the api. The rotation is in radians with 0 being no rotation and grows with a clockwise rotation.
Batch drawing
While you can pretty efficiently draw thousands of objects by calling add() a bunch of times,
you might already have all of the vertex data embeded somewhere, in which case it can seem
wasteful to iterate through your data structure to just build up another list that is then sent
to the gpu. egaku2d has Batches
that lets you map verticies to an existing data structure that you might have.
This lets us skip building up a new verticies list by sending your entire data structure to the gpu.
The downside to this approach is that you might have the vertex data in a list, but it might not be tightly packed since you have a bunch of other data associated with each element, in which case we might end up sending a lot of useless data to the gpu.
Currently this is only supported for circle drawing.
View
The top left corner is the origin (0,0) and x and y grow to the right and downwards respectively.
In windowed mode, the dimenions of the window defaults to scale exactly to the world. For example, if the user made a window of size 800,600, and then drew a circle at 400,300, the circle would appear in the center of the window. Similarily, if the user had a monitor with a resolution of 800,600 and started in fullscreen mode, and drew a circle at 400,300, it would also appear in the center of the screen.
The ratio between the scale of x and y are fixed to be 1:1 so that there is no distortion in the shapes. The user can manually set the scale either by x or y and the other axis is automaically inferred so that to keep a 1:1 ratio.
Fullscreen
Fullscreen is kept behind a feature gate since on certain platforms like wayland linux it does not work. I suspect this is a problem with glutin, so I have just disabled it for the time behing in the hope that once glutin leaves alpha it will work. I think the problem is that when the window is resized, I can't manually change the size of the context to match using resize().
Example
use axgeom::*;
let events_loop = glutin::event_loop::EventLoop::new();
let mut glsys = egaku2d::WindowedSystem::new([600, 480], &events_loop,"test window");
//Make a tileset texture from a png that has 64 different tiles.
let food_texture = glsys.texture("food.png",[8,8]).unwrap();
let canvas = glsys.canvas_mut();
//Make the background dark gray.
canvas.clear_color([0.2,0.2,0.2]);
//Push some squares to a static vertex buffer object on the gpu.
let rect_save = canvas.squares()
.add([40., 40.])
.add([40., 40.])
.save(canvas);
//Draw the squares we saved.
rect_save.uniforms(canvas,5.0).with_color([0.0, 1.0, 0.1, 0.5]).draw();
//Draw some arrows.
canvas.arrows(5.0)
.add([40., 40.], [40., 200.])
.add([40., 40.], [200., 40.])
.send_and_uniforms(canvas).draw();
//Draw some circles.
canvas.circles()
.add([5.,6.])
.add([7.,8.])
.add([9.,5.])
.send_and_uniforms(canvas,4.0).with_color([0., 1., 1., 0.1]).draw();
//Draw some circles from f32 primitives.
canvas.circles()
.add([5.,6.])
.add([7.,8.])
.add([9.,5.])
.send_and_uniforms(canvas,4.0).with_color([0., 1., 1., 0.1]).draw();
//Draw the first tile in the top left corder of the texture.
canvas.sprites().add([100.,100.],food_texture.coord_to_index([0,0]),3.14).send_and_uniforms(canvas,&food_texture,4.0).draw();
//Swap buffers on the opengl context.
glsys.swap_buffers();
Dependencies
~9.5MB
~171K SLoC