7 releases
0.1.6 | May 30, 2023 |
---|---|
0.1.5 | Feb 23, 2021 |
0.0.0 |
|
#3 in #vec2
35 downloads per month
26KB
401 lines
gfxmath-vec2
A simple 2D math library.
Vec2
There are multiple ways we can create a Vec2 object.
use gfxmath_vec2::Vec2;
// Standard 'new' semantics.
let v = Vec2::<f32>::new(3.0, 4.0);
// Using macro 'vec2!'
use gfxmath_vec2::vec2;
let v: Vec2<f32> = vec2!(3.0, 4.0);
// Using 'into' from a tuple.
let v: Vec2<f32> = (3.0, 4.0).into();
// Using 'into' from a borrowed tuple
let t = (3.0, 4.0);
let v: Vec2<f32> = (&t).into();
We can also create tuples from Vec2 objects.
use gfxmath_vec2::Vec2;
// With owned Vec2 object
let v = Vec2::<f32>::new(3.0, 4.0);
let t: (f32, f32) = v.into();
// With borrowed Vec2 object
let v = Vec2::<f32>::new(3.0, 4.0);
let t: (f32, f32) = (&v).into();
Operators
Common mathematical operators are implemented for Vec2.
+
,+=
-
,-=
*
,*=
/
,/=
// Reminder: Rust automatically infers floats to `f64` and integers to `i64`.
use gfxmath_vec2::Vec2;
let v1 = Vec2::new(3.0, 9.0);
let v2 = Vec2::new(4.0, 5.0);
let res = v1 + v2;
assert_eq!(7.0, res.x);
assert_eq!(14.0, res.y);
Known Limitations
Left-Hand Primitives
One caveat is that operators where the Left-Hand side is a primitive has limited support. This is due to restrictions for trait implementations. impl <T> Add<Vec2<T>> for T
is illegal within Rust syntax (due to the trailing T
) because the implementation must be for a known type for non-local traits and types. Since Add
is from the core
package and T
(the type to be implemented for) is not derived from a local trait, this is not possible.
At the time of this writing, primitives that work on the Left-Hand Side for common operators are f32
, f64
, i32
, and i64
.
use gfxmath_vec2::Vec2;
// Works
let lhs: f32 = 4.0;
let rhs = Vec2::<f32>::new(3.0, 4.0);
let res = lhs + rhs;
// NOT SUPPORTED!!
let lhs: u32 = 4;
let rhs = Vec2::<u32>::new(3, 4);
let res = lhs + rhs;
Hash Implementation
Currently, Hash implementations are limited for the following types:
f32
f64
i32
i64
u32
u64
Showcase
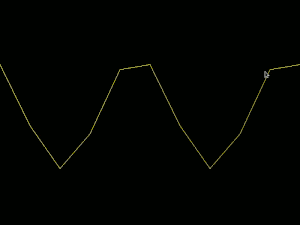
License
Apache 2.0
Dependencies
~210–650KB
~15K SLoC