6 releases (breaking)
0.5.0 | Aug 13, 2024 |
---|---|
0.4.0 | Jun 7, 2024 |
0.3.0 | May 19, 2024 |
0.2.0 | May 9, 2024 |
0.1.1 | Apr 30, 2024 |
#124 in Images
Used in 2 crates
225KB
5.5K
SLoC
auto-palette
🎨 A Rust library for automatically extracting prominent color palettes from images.
Features
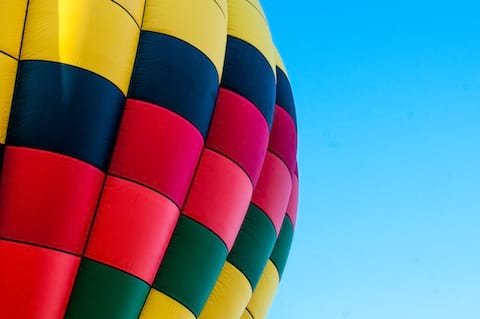

[!NOTE] Photo by Laura Clugston on Unsplash
- Automatically extracts prominent color palettes from images.
- Provides detailed information on color, position, and population.
- Supports multiple extraction algorithms, including
DBSCAN
,DBSCAN++
, andKMeans++
. - Supports multiple color spaces, including
RGB
,HSL
, andLAB
. - Supports the selection of prominent colors based on multiple themes, including
Vivid
,Muted
,Light
, andDark
.
Installation
Using auto-palette
in your Rust project, add it to your Cargo.toml
.
[dependencies]
auto-palette = "0.5.0"
Usage
Here is a basic example that demonstrates how to extract the color palette and find the prominent colors. See the examples directory for more examples.
use auto_palette::{ImageData, Palette};
fn main() {
// Load the image data from the file
let image_data = ImageData::load("../../gfx/holly-booth-hLZWGXy5akM-unsplash.jpg").unwrap();
// Extract the color palette from the image data
let palette: Palette<f64> = Palette::extract(&image_data).unwrap();
println!("Extracted {} swatches", palette.len());
// Find the 5 prominent colors in the palette and print their information
let swatches = palette.find_swatches(5);
for swatch in swatches {
println!("Color: {}", swatch.color().to_hex_string());
println!("Position: {:?}", swatch.position());
println!("Population: {}", swatch.population());
}
}
API
For more information on the API, see the documentation.
ImageData
The ImageData
struct represents the image data that is used to extract the color palette.
ImageData::load
Loads the image data from the file.
The supported image formats are PNG
, JPEG
, GIF
, BMP
, TIFF
, and WEBP
.
This method requires the image
feature to be enabled. The image
feature is enabled by default.
// Load the image data from the file
let image_data = ImageData::load("path/to/image.jpg").unwrap();
ImageData::new
Creates a new instance from the raw image data.
Each pixel is represented by four consecutive bytes in the order of R
, G
, B
, and A
.
// Create a new instance from the raw image data
let pixels = [
255, 0, 0, 255, // Red
0, 255, 0, 255, // Green
0, 0, 255, 255, // Blue
255, 255, 0, 255, // Yellow
];
let image_data = ImageData::new(2, 2, &pixels).unwrap();
Palette
The Palette
struct represents the color palette extracted from the ImageData
.
Palette::extract
Palette::extract_with_algorithm
Palette::find_swatches
Palette::find_swatches_with_theme
Palette::extract
Extracts the color palette from the given ImageData
.
This method is used to extract the color palette with the default Algorithm
(DBSCAN).
// Load the image data from the file
let image_data = ImageData::load("path/to/image.jpg").unwrap();
// Extract the color palette from the image data
let palette: Palette<f64> = Palette::extract(&image_data).unwrap();
Palette::extract_with_algorithm
Extracts the color palette from the given ImageData
with the specified Algorithm
.
The supported algorithms are DBSCAN
, DBSCAN++
, and KMeans++
.
// Load the image data from the file
let image_data = ImageData::load("path/to/image.jpg").unwrap();
// Extract the color palette from the image data with the specified algorithm
let palette: Palette<f64> = Palette::extract_with_algorithm(&image_data, Algorithm::DBSCAN).unwrap();
Palette::find_swatches
Finds the prominent colors in the palette based on the number of swatches.
Returned swatches are sorted by their population in descending order.
// Find the 5 prominent colors in the palette
let swatches = palette.find_swatches(5);
Palette::find_swatches_with_theme
Finds the prominent colors in the palette based on the specified Theme
and the number of swatches.
The supported themes are Basic
, Colorful
, Vivid
, Muted
, Light
, and Dark
.
// Find the 5 prominent colors in the palette with the specified theme
let swatches = palette.find_swatches_with_theme(5, Theme::Light);
Swatch
The Swatch
struct represents the color swatch in the Palette
.
It contains detailed information about the color, position, population, and ratio.
// Find the 5 prominent colors in the palette
let swatches = palette.find_swatches(5);
for swatch in swatches {
// Get the color, position, and population of the swatch
println!("Color: {:?}", swatch.color());
println!("Position: {:?}", swatch.position());
println!("Population: {}", swatch.population());
println!("Ratio: {}", swatch.ratio());
}
[!TIP] The
Color
struct provides various methods to convert the color to different formats, such asRGB
,HSL
, andCIE L*a*b*
.let color = swatch.color(); println!("Hex: {}", color.to_hex_string()); println!("RGB: {:?}", color.to_rgb()); println!("HSL: {:?}", color.to_hsl()); println!("CIE L*a*b*: {:?}", color.to_lab()); println!("Oklch: {:?}", color.to_oklch());
Development
Follow the instructions below to build and test the project:
- Fork and clone the repository.
- Create a new branch for your feature or bug fix.
- Make your changes and write tests.
- Test your changes with
cargo test --lib
. - Format the code with
cargo +nightly fmt
andtaplo fmt
. - Create a pull request.
License
This project is distributed under the MIT License. See the LICENSE file for details.
Dependencies
~5.5MB
~107K SLoC