2 releases
0.1.2 | Aug 10, 2023 |
---|---|
0.1.1 | Jan 14, 2023 |
#1341 in Hardware support
55KB
90 lines
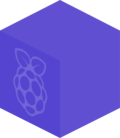
raslib
raslib is a library to manage Raspberry PI devices, written in Rust.
It provides GPIO¹ ports access in order to manage leds or motors (direct support for L298N circuit motors).
Installation
In your "Cargo.toml" file :
[dependencies]
raslib = "*"
Check the current version on crates.io.
Overview
-
GPIO
The library provides a structure to manipulate GPIO ports simply called
Gpio
.#[derive(Copy, Clone)] struct Gpio { pin: u32, }
Implementation
fn new(pin: u32) -> Result<Self, std::io::Error>; fn write(&self, value: bool) -> Result<(), std::io::Error>; fn read(&self) -> Result<bool, std::io::Error>; fn pin(&self) -> u32;
Usage
use raslib::Gpio;
let gpio = Gpio::new(16)?; gpio.write(raslib::HIGH)?; let pin: u32 = gpio.pin();
The Raspberry PI has different GPIO pins following the version. Make sure to connect to the right numbers.
This example is tested on Raspberry PI 4 Model B and the port 16 is not a power (PWR) nor a ground (GND) pin !
See its example.
-
L298N
The library provides a simple way to manipulate motors using the L298N circuit. Because the motors wires are connected to the GPIO pins,
L298n
actually usesGpio
.struct L298n { in1: Gpio, in2: Gpio, ena: Gpio, }
Implementation
fn new(in1: u32, in2: u32, ena: u32) -> Self; fn forward(&mut self) -> Result<(), io::Error>; fn backward(&mut self) -> Result<(), io::Error>; fn stop(&mut self) -> Result<(), io::Error>;
Usage
use raslib::L298n;
let mut motor_left = L298n::new(18, 15, 14); let mut motor_right = L298n::new(9, 7, 25); motor_left.forward()?; motor_right.forward()?;
See its example.
-
Utils
The library provides a simple
sleep
function that makes the current thread wait for a duration in milliseconds.fn sleep(milliseconds: u64);
Usage
raslib::sleep(1000); // waits 1 second.
To make writing values prettier, it also provides two constants:
const HIGH: bool = true; const LOW: bool = false;
Usage
gpio.write(raslib::HIGH); gpio.write(true); // same as above