4 stable releases
1.1.1 | Aug 18, 2023 |
---|---|
1.1.0 | Oct 11, 2022 |
1.0.1 | Oct 10, 2022 |
#105 in Geospatial
124 downloads per month
Used in open-stock
22KB
448 lines
photon-geocoding-rs
An API client for Komoot's Photon API written in and for Rust.
It supports forward and reverse geocoding as well as search-as-you-type.
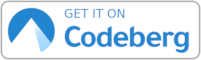
The main repository is hosted on codeberg.org. Issues and Pull Requests are preferred there, but you can still open one on GitHub.
Photon
Photon is a free and open-source API hosted by Komoot and powered by ElasticSearch. It returns data from the OpenStreetMap project, which is licensed under the ODbL License.
The API is available at photon.komoot.io and licensed under the Apache 2.0 License.
Important: Please be aware of the Terms and Use of Photon! It is free to use, so please be fair and avoid excessive requests!
Usage
In your cargo.toml
, include this:
[dependencies]
photon-geocoding = { version = "1.1.0" }
Forward geocoding:
use photon_geocoding::{PhotonApiClient, PhotonFeature};
let api: PhotonApiClient = PhotonApiClient::default();
let result: Vec<PhotonFeature> = api.forward_search("munich", None).unwrap();
Reverse geocoding:
use photon_geocoding::{PhotonApiClient, PhotonFeature};
let api: PhotonApiClient = PhotonApiClient::default();
let result: Vec<PhotonFeature> = api.reverse_search(LatLon::new(48.123, 11.321), None).unwrap();
Self-hosted instances (custom URL):
use photon_geocoding::PhotonApiClient;
let api: PhotonApiClient = PhotonApiClient::new("https://example.com");
// requests will now go to https://example.com/api and https://example.com/reverse
Filters:
use photon_geocoding::filter::{ForwardFilter, PhotonLayer};
use photon_geocoding::{BoundingBox, LatLon, PhotonApiClient};
let api: PhotonApiClient = PhotonApiClient::default();
let filter = ForwardFilter::new()
.language("FR")
.bounding_box(BoundingBox {
south_west: LatLon::new(40.0, 10.0),
north_east: LatLon::new(50.0, 15.0),
})
.layer(vec![PhotonLayer::City, PhotonLayer::State])
.additional_query(vec![("osm_tag", "!key:value")]);
let results = api.forward_search("munich", Some(filter)).unwrap();
// resulting query string: "q=munich&bbox=10%2C40%2C15%2C50&lang=fr&layer=city&layer=state&osm_tag=%21key%3Avalue"
All requests are performed in blocking mode, so no async behavior is involved. However, the PhotonApiClient
is thread-safe, so you can safely choose to do multiple requests in parallel using the same instance.
Features and Bugs
Feel free to open a new issue! I am always happy to improve this package.
As I am fairly new to Rust, please also don't hesitate to suggest improvements on code style and/or usability (especially regarding ownership, borrowing etc.)!
Contribution
Feel free to open pull requests and help to improve this package!
Dependencies
~5MB
~87K SLoC