4 stable releases
1.3.4 | Aug 5, 2020 |
---|---|
1.3.2 | Aug 4, 2020 |
1.3.1 | Aug 3, 2020 |
#153 in Profiling
455 downloads per month
Used in 9 crates
(3 directly)
6MB
251 lines
Contains (static library, 3.5MB) external/lib/x64/OptickCore_MT.lib, (static library, 2.5MB) external/lib/x64/OptickCore_MD.lib
Rust API for Optick Profiler
Supported Platforms
Windows (x64) | Linux | MacOS |
---|---|---|
YES | NO (IN PROGRESS) | NO (IN PROGRESS) |
How to use
In Cargo.toml
add:
[dependencies]
optick = "1.3.4"
Example 1 (generic app, automation, etc.):
fn calc(n: u32) {
// Profile current scope (automatically extracts current function name)
// You could also specify a custom name if needed - e.g. optick::scope!("calc");
optick::event!();
// Attach custom data tag to the capture (i32, u32, u64, f32, str, vec3)
optick::tag!("number", n);
optick::tag!("name", "Bob");
optick::tag!("position", (10.0f32, -12.0f32, 14.0f32));
...
}
pub fn main() {
// Start a new capture
optick::start_capture();
calc(42);
// Stop and save current capture to {working_dir}/capture_name(date-time).opt
optick::stop_capture("capture_name");
}
Example 2 (game):
fn update(frame_num: u32) {
optick::event!();
optick::tag!("frame", frame_num);
std::thread::sleep(std::time::Duration::from_millis(33));
}
pub fn main() {
let mut frame = 0;
loop {
optick::next_frame();
update(frame);
frame = frame + 1;
}
}
GUI
Use Optick GUI to open saved *.opt capture for further analysis:
https://github.com/bombomby/optick/releases
After grabbing the latest available Optick_vX.X.X.zip => launch Optick.exe.
Video Tutorial (Features Overview)
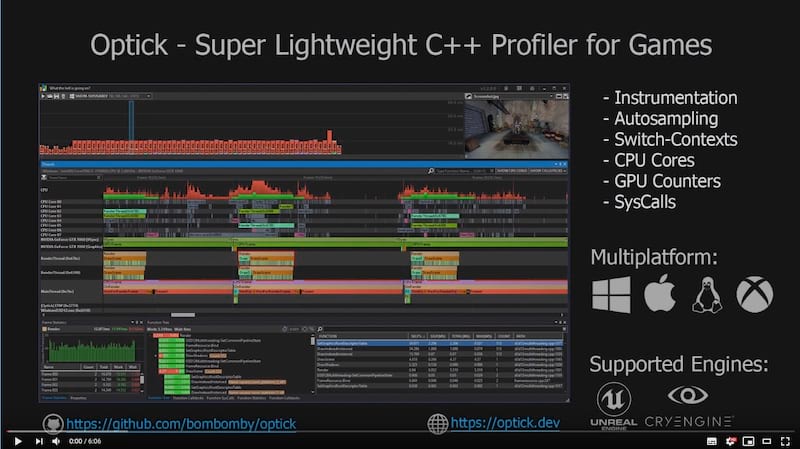
Procedural Macros
Optick supports a set of procedural macros for simplifying the process of code markup: https://crates.io/crates/optick-attr
// Instrument current function
#[optick_attr::profile]
fn calc() {
// Do some stuff
}
//Generate performance capture for function to {working_dir}/capture_name(date-time).opt.
#[optick_attr::capture("capture_name")]
pub fn main() {
calc();
}
Feature flags
enable
- this flag is used by default and enables Optick instrumentation
Run as Administartor to collect ETW events
Optick uses ETW to collect hardware counters: switch-contexts, auto-sampling, CPU core utilization, etc. Run your app as administrator to enable the collection of ETW events:
Start-Process cargo run -Verb runAs