44 releases
0.8.9 | Jun 21, 2024 |
---|---|
0.8.8 | Nov 15, 2023 |
0.8.6 | Jul 25, 2023 |
0.8.5 | Oct 17, 2022 |
0.0.8 | Dec 16, 2017 |
#162 in HTTP server
79 downloads per month
1.5MB
3.5K
SLoC
http-server
Simple and configurable command-line HTTP server
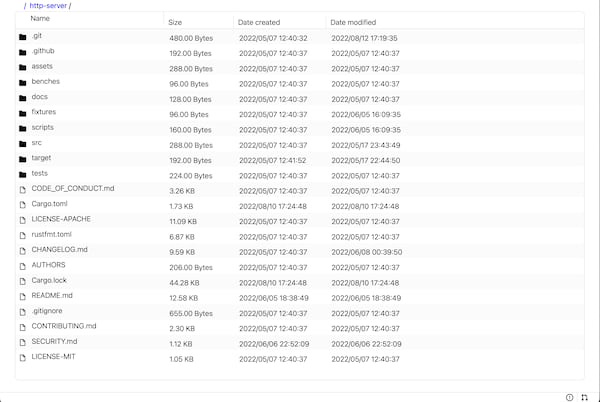
Installation
cargo install http-server
Verify successful installation.
http-server --help
Expect the following output:
USAGE:
http-server [FLAGS] [OPTIONS] [root-dir]
FLAGS:
--cors Enable Cross-Origin Resource Sharing allowing any origin
--graceful-shutdown Waits for all requests to fulfill before shutting down the server
--gzip Enable GZip compression for HTTP Responses
--help Prints help information
-l, --logger Prints HTTP request and response details to stdout
-q, --quiet Turns off stdout/stderr logging
--spa Route non-existent files to /index.html
--tls Enables HTTPS serving using TLS
-i, --index Route directories to index.html if present
-V, --version Prints version information
OPTIONS:
-c, --config <config> Path to TOML configuration file
-h, --host <host> Host (IP) to bind the server [default: 127.0.0.1]
--password <password> Specifies password for basic authentication
-p, --port <port> Port to bind the server [default: 7878]
--proxy <proxy> Proxy requests to the provided URL
--tls-cert <tls-cert> Path to the TLS Certificate [default: cert.pem]
--tls-key <tls-key> Path to the TLS Key [default: key.rsa]
--tls-key-algorithm <tls-key-algorithm> Algorithm used to generate certificate key [default: rsa]
--username <username> Specifies username for basic authentication
ARGS:
<root-dir> Directory to serve files from [default: ./]
If you find this output is out of date, don't hesitate to open a PR here.
Configuration
When running the server with no options or flags provided, a set of default configurations will be used. You can always change this behavior by either creating your own config with the Configuration TOML file or by providing CLI arguments described in the usage section.
Name | Description | Default |
---|---|---|
Host | Address to bind the server | 127.0.0.1 |
Port | Port to bind the server | 7878 |
Root Directory | The directory to serve files from | CWD |
File Explorer UI | A File Explorer UI for the directory configured as the Root Directory | Enabled |
Configuration File | Specifies a configuration file. Example | Disabled |
HTTPS (TLS) | HTTPS Secure connection configuration. Refer to TLS (HTTPS) reference | Disabled |
CORS | Cross-Origin-Resource-Sharing headers support. Refer to CORS reference | Disabled |
Compression | GZip compression for HTTP Response Bodies. Refer to Compression reference | Disabled |
Quiet | Don't print server details when running. This doesn't include any logging capabilities. | Disabled |
Index | Route directories to index.html if present | Disabled |
SPA | Route non-existent files to /index.html | Disabled |
Basic Authentication | Authorize requests using Basic Authentication. Refer to Basic Authentication | Disabled |
Logger | Prints HTTP request and response details to stdout | Disabled |
Usage
http-server [FLAGS] [OPTIONS] [root-dir]
Flags
Flags are provided without any values. For example:
http-server --help
Name | Short | Long | Description |
---|---|---|---|
Cross-Origin Resource Sharing | N/A | --cors |
Enable Cross-Origin Resource Sharing allowing any origin |
GZip Compression | N/A | --gzip |
Enable GZip compression for responses |
Graceful Shutdown | N/A | --graceful-shutdown |
Wait for all requests to be fulfilled before shutting down the server |
Help | N/A | --help |
Print help information |
Logger | -l |
--logger |
Print HTTP request and response details to stdout |
Version | -V |
--version |
Print version information |
Quiet | -q |
--quiet |
Don't print output to console |
Index | -i |
--index |
Route directories to index.html if present |
SPA | N/A | --spa |
Route non-existent files to /index.html |
Options
Options receive a value and support default values as well.
http-server --host 127.0.0.1
Name | Short | Long | Description | Default Value |
---|---|---|---|---|
Host | -h |
--host |
Address to bind the server | 127.0.0.1 |
Port | -p |
--port |
Port to bind the server | 7878 |
Configuration File | -c |
--config |
Configuration file. Example | N/A |
TLS | N/A | --tls |
Enable TLS for HTTPS connections. Requires a Certificate and Key. Reference | N/A |
TLS Certificate | N/A | --tls-cert |
Path to TLS certificate file. Depends on --tls |
cert.pem |
TLS Key | N/A | --tls-key |
Path to TLS key file. Depends on --tls |
key.rsa |
TLS Key Algorithm | N/A | --tls-key-algorithm |
Algorithm used to generate certificate key. Depends on --tls |
rsa |
Username | N/A | --username |
Username to validate using basic authentication | N/A |
Password | N/A | --password |
Password to validate using basic authentication. Depends on --username |
N/A |
Proxy | N/A | --proxy |
Proxy requests to the provided URL | N/A |
Request Handlers
This HTTP Proxy supports different Request Handlers which determine how each incoming HTTP request is handled. They can't be combined, you must choose one based on your needs.
- File Server default
- Proxy
File Server Handler
Serves files from the provided directory. Navigation is scoped to the specified directory. If no directory is provided the CWD will be used.
This is the default behavior for the HTTP server.
Proxy Handler
Proxies requests to the provided URL. The URL provided is used as the base URL for incoming requests.
Reference
The following are some relevant details on features supported by this HTTP Server that may be of interest to the user.
Compression
Even though compression is supported, by default the server will not compress any HTTP response contents. You must specify the compression configuration you want to use, in the configuration file or on the command line.
As of today the server only supports compression with the GZip algorithm, but
brotli
support is also planned.
The following MIME types are never compressed:
application/gzip
application/octet-stream
application/wasm
application/zip
image/*
video/*
The Configuration File's Compression Section
As future support for other compression algorithms is planned, the configuration file already supports compression settings.
[compression]
gzip = true
The --gzip
flag
Provide the --gzip
argument to the server when executing it.
http-server --gzip
TLS (HTTPS)
The TLS solution supported for this HTTP Server is built with the rustls crate along with hyper-rustls.
When running with TLS support you will need:
- A certificate
- A matching RSA Private Key for the certificate
A script to generate certificates and keys is available here tls-cert.sh.
This script relies on openssl
, so make sure you have it installed on your system.
Run http-server
as follows:
http-server --tls --tls-cert <PATH TO YOUR CERTIFICATE> --tls-key <PATH TO YOUR KEY> --tls-key-algorithm pkcs8
Cross-Origin Resource Sharing (CORS)
This HTTP Server supports CORS headers out of the box. Based on the headers you want to provide in your HTTP Responses, two different methods for CORS configuration are available.
By providing the --cors
option to http-server
, CORS headers
will be appended to every HTTP Response, allowing any origin.
For more complex configurations, like specifying an origin, a set of allowed HTTP methods and more, you should specify the configuration via the configuration TOML file.
The following example shows all the available options.
[cors]
allow_credentials = false
allow_headers = ["content-type", "authorization", "content-length"]
allow_methods = ["GET", "PATCH", "POST", "PUT", "DELETE"]
allow_origin = "example.com"
expose_headers = ["*", "authorization"]
max_age = 600
request_headers = ["x-app-version"]
request_method = "GET"
Basic Authentication
Basic Authentication is supported to deny requests when credentials are invalid.
You must provide the allowed username
and password
either by using the CLI
options --username
along with the desired username and --password
along with
the desired password, or by specifying such values through the configuration
TOML file.
[basic_auth]
username = "John"
password = "Appleseed"
Proxy
The HTTP Server is able to proxy requests to a specified URL.
When using the proxy, the FileExplorer won't be available, as the proxy is an alternate Request Handler.
The config TOML file can be used to provide proxy configurations:
[proxy]
url = "https://example.com"
Roadmap
The following roadmap list features to provide for the version v1.0.0
.
This roadmap is still open for suggestions. If you find that there's a missing feature in this list, that you would like to work on or expect for the first stable release, please contact the software editors by opening an issue or a discussion.
If you want to contribute to one of these, please make sure there's an issue tracking the feature and ping me. Otherwise open an issue to be assigned and track the progress there.
- Logging
- Request/Response Logging
- Service Config Logins
- File Explorer
- Modified Date
- File Size
- Breadcrumb Navigation
- File Upload
- Filtering
- Sorting
- Sort By: File Name
- Sort By: File Size
- Sort By: File Modified Date
- Directories First
- Files First
- HTTPS/TLS Serving
- HTTPS/TLS Support
- Compression
-
gzip/deflate
Compression -
brotli
Compression
-
- CORS
- Cross Origin Resource Sharing
- Allow Credentials
- Allow Headers
- Allow Methods
- Allow Origin
- Expose Headers
- Max Age
- Request Headers
- Request Methods
- Multiple Origins (#8)
- Cross Origin Resource Sharing
- Cache Control
-
Last-Modified
andETag
- Respond with 304 to
If-Modified-Since
-
- Partial Request
-
Accept-Ranges
-
Content-Range
-
If-Range
-
If-Match
-
Range
-
- Standalone Builds
- macOS
- Linux
- Windows
- Development Server
- Live Reload
- Proxy
- URL Configuration
- Basic Authentication
- Username
- Password
- Graceful Shutdown
Release
In order to create a release you must push a Git tag as follows
git tag -a <version> -m <message>
Example
git tag -a v0.1.0 -m "First release"
Tags must follow semver conventions. Tags must be prefixed with a lowercase
v
letter.
Then push tags as follows:
git push origin main --follow-tags
Contributing
Every contribution to this project is welcome. Feel free to open a pull request or an issue. Just by using this project you're helping it grow. Thank you!
License
Distributed under the terms of both the MIT license and the Apache License (Version 2.0)
Dependencies
~19–32MB
~563K SLoC