2 unstable releases
0.2.0 | Oct 8, 2020 |
---|---|
0.1.0 | Jul 21, 2020 |
#27 in #example
13KB
174 lines
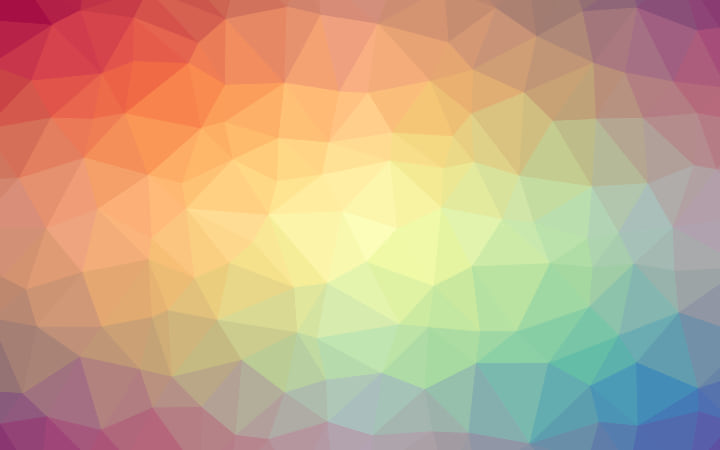
Code Tour
Introduction
This project is an attempt to improve Rust example-based learning approach.
Imagine the following example file:
#[derive(Debug)]
struct S {
x: i32,
y: i32,
}
fn main() {
// Declare something.
// Because it's an example.
let a = S { x: 7, y: 42 };
let b = a.x + a.y;
// Here is the result!
let c = b + 1;
}
When one runs the example with cargo run --example foo
, nothing is
printed! It means the author of the example must add println!
or
dbg!
instructions everytime. Moreover the users are going to miss
the comments, that's really unfortunate.
Enter code_tour
.
Let's rewrite the example as such:
use code_tour::code_tour;
#[derive(Debug)]
struct S {
x: i32,
y: i32,
}
#[code_tour]
fn main() {
/// Declare something.
/// Because it's an example.
let a = S { x: 7, y: 42 };
let b = a.x + a.y;
/// Here is the result!
let c = b + 1;
}
Let's re-execute the example as previously, and we'll see:
$ cargo run --example foo
The example annotations are replicated on the output during the execution.
An annotation must be a comment of kind ///
or /** … */
that
introduces a let
binding. That's all for the moment!
Interactive mode
Running the example with --features interactive
will display a
“Press Enter to continue, otherwise Ctrl-C to exit.” message after
each step of your code.
$ cargo run --example foo --features interactive
Quiet mode
Running the example with the environment variable CODE_TOUR_QUIET
set will turn code-tour silent. Note that it won't disable the
interactive mode (which is on purpose).
$ CODE_TOUR_QUIET=1 cargo run --example foo
Better source code display
Running the example with cargo +nightly
will generate a better
output for the code, by using
Span::source_text
.
$ cargo +nightly run --example foo
Install
This is a classic Rust project, thus add code_tour
to your
Cargo.toml
file, and that's it.
License
BSD-3-Clause
, see LICENSE.md
.
Dependencies
~1.3–1.7MB
~38K SLoC