8 releases (stable)
1.4.1 | Nov 19, 2023 |
---|---|
1.4.0 | Nov 17, 2023 |
1.3.2 | Sep 30, 2023 |
1.2.0 | Aug 3, 2023 |
0.1.0 | Aug 1, 2023 |
#1675 in Web programming
38 downloads per month
540KB
204 lines
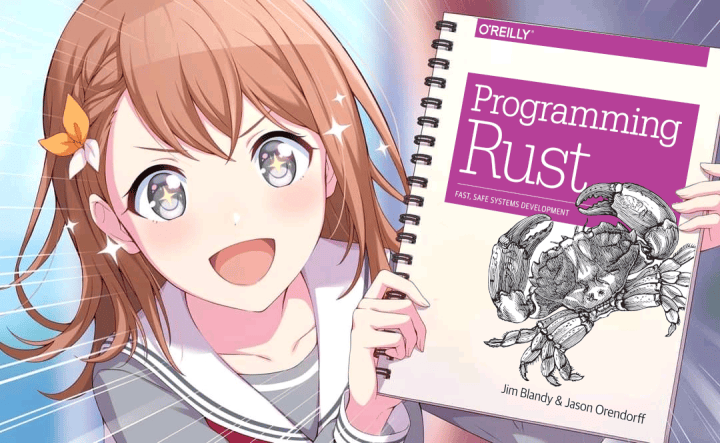
[!Note]
This is part of my aghpb api wrapper challenge where I attempt to write an api wrapper in every language possible. So yes expect spaghetti code as it will be my first time writing in these languages. Although I'm 100% open to improvements and corrections so feel free to contribute anything. Other languages I've done
Install
cargo add aghpb
More install instructions at crates.io.
Examples
This is how you may retrieve a random anime girls holding programming books:
use tokio::fs;
use std::error::Error;
#[tokio::main]
async fn main() -> Result<(), Box<dyn Error>> {
let book = aghpb::random(None).await?;
println!("Name: {}", book.name);
println!("Category: {}", book.category);
println!("Date added: {}", book.date_added);
fs::write("./anime_girl.png", book.raw_bytes).await?;
Ok(())
}
You can also retrieve specific categories of anime girls holding programming books like so:
let book = aghpb::random(Some("rust".into())).await?;
This is how you may retrieve a list of available categories:
use std::error::Error;
#[tokio::main]
async fn main() -> Result<(), Box<dyn Error>> {
let categories = aghpb::categories().await?;
for category in categories {
println!("{}", category);
}
Ok(())
}
How to search for an anime girl holding a programming book.
[!NOTE] NEW in v1.4!
use std::error::Error;
use tokio::fs;
#[tokio::main]
async fn main() -> Result<(), Box<dyn Error>> {
let books = aghpb::search("tohru".into(), None, None).await?;
let book_data = &books[0]; // I'm selecting the first book just for this example.
println!("Name: {}", book_data.name);
println!("Category: {}", book_data.category);
println!("Commit Author: {}", book_data.commit_author);
println!("Commit URL: {}", book_data.commit_url);
println!("Date Added: {}", book_data.date_added);
println!("Search ID: {}", book_data.search_id);
let book = book_data.get_book().await?;
fs::write("./anime_girl.png", book.raw_bytes).await?;
Ok(())
}
Made using my API at 👉 https://api.devgoldy.xyz/aghpb/v1/
Dependencies
~4–17MB
~245K SLoC