36 stable releases
new 3.0.3 | Mar 28, 2025 |
---|---|
3.0.1 | Feb 25, 2025 |
2.1.1 | Feb 13, 2025 |
1.2.3 | Jan 24, 2025 |
1.0.14 | Nov 27, 2023 |
#145 in Algorithms
315 downloads per month
90KB
1.5K
SLoC
Supercluster
A high-performance Rust crate for geospatial and non-geospatial point clustering.
Reference implementation
Documentation
For more in-depth details, please refer to the full documentation.
If you encounter any issues or have questions that are not addressed in the documentation, feel free to submit an issue.
This crate was initially inspired by Mapbox's supercluster blog post.
Usage
To use the supercluster
crate in your project, add it to your Cargo.toml
:
[dependencies]
supercluster = "3.0.0"
You can also include additional features, such as logging, by specifying them in your Cargo.toml
:
[dependencies]
supercluster = { version = "3.0.0", features = ["log", "serde", "cluster_metadata"] }
Below is an example of how to create and run a supercluster using the crate. This example demonstrates how to build supercluster options, create a new supercluster, and get a tile. For more detailed information and advanced usage, please refer to the full documentation.
use supercluster::{ CoordinateSystem, Supercluster, SuperclusterError };
fn main() -> Result<(), SuperclusterError> {
// Set the configuration settings
let options = Supercluster::builder()
.radius(40.0)
.extent(512.0)
.min_points(2)
.max_zoom(16)
.coordinate_system(CoordinateSystem::LatLng)
.build();
// Create a new instance with the specified configuration settings
let mut cluster = Supercluster::new(options);
// Create a a list of features
let features = Supercluster::feature_builder()
.add_point(vec![0.0, 0.0])
.build();
// Load a list of features into the supercluster
let index = cluster.load(features)?;
index.get_tile(0, 0.0, 0.0)?;
Ok(())
}
Benchmarks
We use the criterion
crate to benchmark the performance of the supercluster
crate.
Benchmarks help us understand the performance characteristics of supercluster and identify areas for optimization.
We have several benchmark scenarios to test different aspects of supercluster:
- Getting a Tile: Tests the performance of retrieving a tile from the
Supercluster
. - Getting Clusters: Tests the performance of retrieving clusters for a given bounding box and zoom level.
- Loading a Feature Collection: Tests the performance of loading a
FeatureCollection
into theSupercluster
.
For more detailed benchmark scenarios, please refer to the benches
directory in the repository.
Safety
This crate uses #![forbid(unsafe_code)]
to ensure everything is implemented in 100% safe Rust.
Contributing
🎈 Thanks for your help improving the project! We are so happy to have you!
We have a contributing guide to help you get involved in the project.
Sponsors
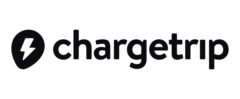
Dependencies
~1.8–2.8MB
~57K SLoC