2 unstable releases
0.2.0 | Jan 23, 2024 |
---|---|
0.1.0 | Jan 13, 2024 |
#38 in #xlsx
470 downloads per month
Used in 3 crates
(via rust_xlsxwriter)
47KB
426 lines
rust_xlsxwriter_derive
The rust_xlsxwriter_derive
crate provides the XlsxSerialize
derived
trait which is used in conjunction with rust_xlsxwriter
serialization.
XlsxSerialize
can be used to set container and field attributes for structs to
define Excel formatting and other options when serializing them to Excel using
rust_xlsxwriter
and Serde
.
use rust_xlsxwriter::{Workbook, XlsxError, XlsxSerialize};
use serde::Serialize;
fn main() -> Result<(), XlsxError> {
let mut workbook = Workbook::new();
// Add a worksheet to the workbook.
let worksheet = workbook.add_worksheet();
// Create a serializable struct.
#[derive(XlsxSerialize, Serialize)]
#[xlsx(header_format = Format::new().set_bold())]
struct Produce {
#[xlsx(rename = "Item", column_width = 12.0)]
fruit: &'static str,
#[xlsx(rename = "Price", num_format = "$0.00")]
cost: f64,
}
// Create some data instances.
let items = [
Produce {
fruit: "Peach",
cost: 1.05,
},
Produce {
fruit: "Plum",
cost: 0.15,
},
Produce {
fruit: "Pear",
cost: 0.75,
},
];
// Set the serialization location and headers.
worksheet.set_serialize_headers::<Produce>(0, 0)?;
// Serialize the data.
worksheet.serialize(&items)?;
// Save the file to disk.
workbook.save("serialize.xlsx")?;
Ok(())
}
The output file is shown below. Note the change or column width in Column A, the renamed headers and the currency format in Column B numbers.
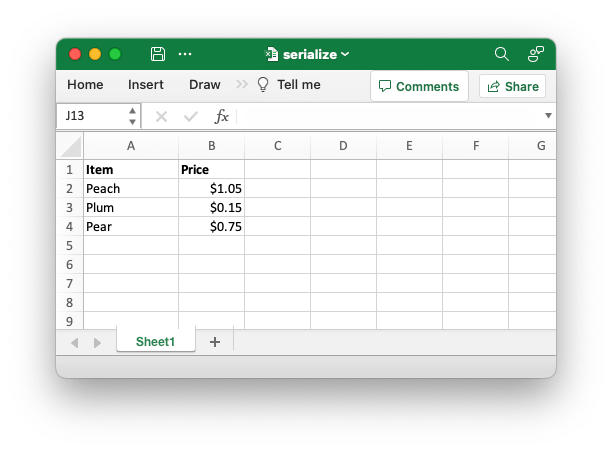
For more information see the documentation on Working with Serde in the
rust_xlsxwriter
docs.
See also
Dependencies
~300–750KB
~18K SLoC