10 releases
0.1.9 | Nov 9, 2024 |
---|---|
0.1.8 | Nov 9, 2024 |
#435 in Math
753 downloads per month
1.5MB
19K
SLoC
ROOC
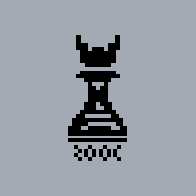
Optimization modeling language
Go to the language documentation
Go to the library documentation
ROOC stands for the courses I took in university—Ricerca Operativa (Operational Research) and Ottimizzazione Combinatoria (Combinatorial Optimization)—which deal with solving optimization models.
What it is
ROOC is a compiler designed to parse and convert formal optimization models into static formulations. These static formulations can be transformed into linear models which can then be solved using optimization techniques.
The language provides support for defining formal models, including functions, constants, arrays, graphs, tuples, etc... It also includes built-in utility functions for iterating over graphs, edges, arrays, ranges, and more.
The library is compiled as a WebAssembly (WASM) module and integrated into the web editor, which features Language Server Protocol (LSP) support for type checking, code completion, and documentation.
Examples
For examples of using the rust lib look at the examples folder
For examples of models look in the rooc docs
Solvers
Currently in ROOC you can solve any linear models which can be:
- MILP
- Integer or binary only
- Binary only
- Real only
Implemented Features
- Language
- Static block functions (min, max, mod, avg)
- Constant Graph definitions
- Iterators
- Tuples
- Iterators utility functions (for graphs, edges, etc)
- Primitive destructuring
- Constants and multi dimensional arrays in the formal definition of a problem
- Other utility functions
- Error logging and parameter validation
- Error traces
- Primitives Operator overloading (for example,
+
for strings) - Definition of variable bounds
- Javascript defined functions, define js functions to use in the model
- Simplex resolution
- Linearization of a generic problem (done except for mod operator)
- Transformation of a linear problem into the standard form
- Two step method using artifical variables to find a valid basis for the standard form problem
- Simplex to find the optimal solution of a standard form linear problem
- Integer and binary problems resolution
- Integer and binary problem definitions (bounds)
- Integer solvers
- Binary problem solution
- Integer/Binary problem solution
- MILP problem solution
- Logic constraints
- UI
- Compilation to WASM
- Create and manage your models
- Automatic compilation to a LATEX block
- LSP
- Syntax errors
- Hover types
- Type errors
- Code completion
- Language documentation
- Show the different steps of solving the problem
- List of modifications from the start of the problem to the end of the solution
Example
Given the formal model of the Dominating set problem, which shows most of the features of the language:
min sum(u in nodes(G)) { x_u }
s.t.
x_v + sum((_, u) in neigh_edges(v)) { x_u } >= 1 for v in nodes(G)
where
let G = Graph {
A -> [B, C, D, E, F],
B -> [A, E, C, D, J],
C -> [A, B, D, E, I],
D -> [A, B, C, E, H],
E -> [A, B, C, D, G],
F -> [A, G, J],
G -> [E, F, H],
H -> [D, G, I],
I -> [C, H, J],
J -> [B, F, I]
}
define
x_u, x_v as Boolean for v in nodes(G), (_, u) in neigh_edges(v)
It is compiled down to:
min x_A + x_B + x_C + x_D + x_E + x_F + x_G + x_H + x_I + x_J
s.t.
x_A + x_B + x_D + x_C + x_F + x_E >= 1
x_B + x_D + x_E + x_J + x_C + x_A >= 1
x_C + x_B + x_D + x_I + x_A + x_E >= 1
x_D + x_E + x_H + x_C + x_A + x_B >= 1
x_E + x_B + x_D + x_C + x_A + x_G >= 1
x_F + x_J + x_G + x_A >= 1
x_G + x_E + x_F + x_H >= 1
x_H + x_D + x_I + x_G >= 1
x_I + x_J + x_H + x_C >= 1
x_J + x_F + x_I + x_B >= 1
If the compilation finds a type mismatch (for example, function parameters or compound variable flattening), a stack trace will be generated:
Wrong argument Expected argument of type "Number", got "Graph" evaluating "D"
at 3:30 D
at 3:28 C[D]
at 3:18 enumerate(C[D])
at 3:9 sum(j in enumerate(C[D])) { j }
at 3:9 sum(j in enumerate(C[D])) { j } <= x_i for i in 0..len(C)
The model can then be solved using the Binary solver
pipeline, which will solve the compiled model and find the optimal solution which has value 3
with assignment:
F F F F T F F F T T
Dependencies
~15MB
~275K SLoC