15 releases (breaking)
0.12.0 | Feb 15, 2022 |
---|---|
0.11.0 | Oct 3, 2021 |
0.10.0 | Jul 28, 2021 |
0.6.2 | Mar 8, 2021 |
0.1.0 | Jul 31, 2020 |
#504 in HTTP server
35KB
402 lines
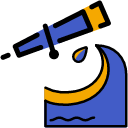
opentelemetry-tide
OpenTelemetry integration for Tide
Add OpenTelemetry tracing and metrics support to your tide application. Be part of the new observability movement!
Notes
- It only implements very basic request tracing on the middleware layer. If you need spans for your executed code, you need to add them yourself.
- It provides basic prometheus metrics, based on the RED method.
- This project got inspired by https://github.com/OutThereLabs/actix-web-opentelemetry.
- You probably do not want to use it in production. 🤷
How to use
# Run jaeger in background
docker run -d \
-p6831:6831/udp -p6832:6832/udp -p16686:16686 -p14268:14268 \
jaegertracing/all-in-one:latest
# Run server example with tracing middleware
cargo run --example server
# Make a request or two ...
curl http://localhost:3000/
# Open browser and view the traces
firefox http://localhost:16686/
# Check the prometheus metrics endpoint
curl http://localhost:3000/metrics
Example
Cargo.toml
# ...
[dependencies]
async-std = { version = "1.10", features = ["attributes"] }
opentelemetry = { version = "0.17.0", features = ["rt-async-std"] }
opentelemetry-jaeger = { version = "0.16.0", features = ["rt-async-std"] }
opentelemetry-tide = "0.12"
tide = "0.16"
server.rs
use opentelemetry::{global, KeyValue, runtime};
use opentelemetry_semantic_conventions::resource;
use opentelemetry_tide::TideExt; // import trait
const VERSION: &'static str = env!("CARGO_PKG_VERSION");
#[async_std::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
tide::log::with_level(tide::log::LevelFilter::Warn);
let tags = [resource::SERVICE_VERSION.string(VERSION)];
let _tracer = opentelemetry_jaeger::new_pipeline()
.with_tags(tags.iter().map(ToOwned::to_owned))
.install_batch(runtime::AsyncStd)
.expect("pipeline install failure");
let tracer = global::tracer("example-server");
let metrics_kvs = vec![KeyValue::new("K", "V")];
let mut app = tide::new();
// use the trait
app.with_middlewares(tracer, Some(metrics_kvs));
app.at("/").get(|_| async move {
Ok("Hello, OpenTelemetry!")
});
app.listen("0.0.0.0:3000").await?;
global::shutdown_tracer_provider();
Ok(())
}
Cargo Features
flag | description |
---|---|
trace |
enables tracing middleware; enabled by default via full |
metrics |
enables metrics middleware; enabled by default via full |
full |
includes both trace and metrics features, enabled by default |
Safety
This crate uses #![forbid(unsafe_code)]
to ensure everything is implemented in 100% Safe Rust.
License
Licensed under either of Apache License, Version 2.0 or MIT license at your option.Unless you explicitly state otherwise, any contribution intentionally submitted for inclusion in this crate by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any additional terms or conditions.
Dependencies
~12–22MB
~329K SLoC