43 releases (16 stable)
1.7.2 | May 13, 2022 |
---|---|
1.7.0 | Mar 12, 2022 |
1.6.0 | Nov 13, 2021 |
1.4.0 | May 14, 2021 |
0.8.1 | Nov 26, 2019 |
#82 in HTTP client
360,334 downloads per month
Used in 292 crates
(128 directly)
365KB
6K
SLoC
Isahc
Say hello to Isahc (pronounced like Isaac), the practical HTTP client that is fun to use.
Formerly known as chttp.
Key features
- Full support for HTTP/1.1 and HTTP/2.
- Configurable request timeouts, redirect policies, Unix sockets, and many more settings.
- Offers an ergonomic synchronous API as well as a runtime-agnostic asynchronous API with support for async/await.
- Fully asynchronous core, with incremental reading and writing of request and response bodies and connection multiplexing.
- Sessions and cookie persistence.
- Automatic request cancellation on drop.
- Uses the http crate as an interface for requests and responses.
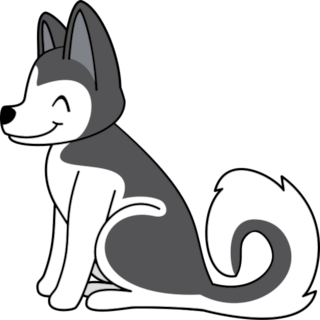
What is Isahc?
Isahc is an acronym that stands for Incredible Streaming Asynchronous HTTP Client, and as the name implies, is an asynchronous HTTP client for the Rust language. It uses libcurl as an HTTP engine inside, and provides an easy-to-use API on top that integrates with Rust idioms.
No, who is Isahc?
Oh, you mean Isahc the dog! He's an adorable little Siberian husky who loves to play fetch with webservers every day and has a very cURLy tail. He shares a name with the project and acts as the project's mascot.
You can pet him all day if you like, he doesn't mind. Though, he prefers it if you pet him in a standards-compliant way!
Documentation
Please check out the documentation for details on what Isahc can do and how to use it. To get you started, here is a really simple, complete example that spits out the response body from https://example.org:
use isahc::prelude::*;
fn main() -> Result<(), isahc::Error> {
// Send a GET request and wait for the response headers.
// Must be `mut` so we can read the response body.
let mut response = isahc::get("https://example.org")?;
// Print some basic info about the response to standard output.
println!("Status: {}", response.status());
println!("Headers: {:#?}", response.headers());
// Read the response body as text into a string and print it.
print!("{}", response.text()?);
Ok(())
}
Click here for documentation on the latest version. You can also click here for built documentation from the latest unreleased master
build.
Getting help
Need some help with something Isahc-related? Ask a question on our discussions page, where we are happy to try and answer your questions!
Installation
Install via Cargo by adding to your Cargo.toml
file:
[dependencies]
isahc = "1.7"
Minimum supported Rust version
The minimum supported Rust version (or MSRV) for Isahc is stable Rust 1.46 or greater, meaning we only guarantee that Isahc will compile if you use a rustc version of at least 1.46. It might compile with older versions but that could change at any time.
This version is explicitly tested in CI and may only be bumped in new minor versions. Any changes to the supported minimum version will be called out in the release notes.
Project goals
- Create an ergonomic and innovative HTTP client API that is easy for beginners to use, and flexible for advanced uses.
- Provide a high-level wrapper around libcurl.
- Maintain a lightweight dependency tree and small binary footprint.
- Provide additional client features that may be optionally compiled in.
Non-goals:
- Support for protocols other than HTTP.
- Alternative engines besides libcurl. Other projects are better suited for this.
Why use Isahc and not X?
Isahc provides an easy-to-use, flexible, and idiomatic Rust API that makes sending HTTP requests a breeze. The goal of Isahc is to make the easy way also provide excellent performance and correctness for common use cases.
Isahc uses libcurl under the hood to handle the HTTP protocol and networking. Using curl as an engine for an HTTP client is a great choice for a few reasons:
- It is a stable, actively developed, and very popular library.
- It is well-supported on a diverse list of platforms.
- The HTTP protocol has a lot of unexpected gotchas across different servers, and curl has been around the block long enough to handle many of them.
- It is well optimized and offers the ability to implement asynchronous requests.
Safe Rust bindings to libcurl are provided by the curl crate, which you can use yourself if you want to use curl directly. Isahc delivers a lot of value on top of vanilla curl, by offering a simpler, more idiomatic API and doing the hard work of turning the powerful multi interface into a futures-based API.
When would you not use Isahc?
Not every library is perfect for every use-case. While Isahc strives to be a full-featured and general-purpose HTTP client that should work well for many projects, there are a few scenarios that Isahc is not well suited for:
- Tiny binaries: If you are creating an application where tiny binary size is a key priority, you might find Isahc to be too large for you. While Isahc's dependencies are carefully curated and a number of features can be disabled, Isahc's core feature set includes things like async which does have some file size overhead. You might find something like ureq more suitable.
- WebAssembly support: If your project needs to be able to be compiled to WebAssembly, then Isahc will probably not work for you. Instead you might like an HTTP client that supports multiple backends such as Surf.
- Rustls support: We hope to support rustls as a TLS backend someday, it is not currently supported directly. If for some reason rustls is a hard requirement for you, you'll need to use a different HTTP client for now.
Sponsors
Special thanks to sponsors of my open-source work!

License
This project's source code and documentation are licensed under the MIT license. See the LICENSE file for details.
The Isahc logo and related assets are licensed under a Creative Commons Attribution 4.0 International License. See LICENSE-CC-BY for details.
Dependencies
~12–24MB
~398K SLoC