18 releases
0.0.18 | Oct 29, 2024 |
---|---|
0.0.17 | Oct 18, 2024 |
0.0.16 | Aug 18, 2024 |
0.0.11 | Jul 30, 2024 |
0.0.1 | Feb 26, 2024 |
#338 in Math
125KB
199 lines
Impact-rs
This crate provides utilities for performing collision queries between rectangles and rays,
including swept checks for moving rectangles. It leverages fixed-point arithmetic provided by the fixed32
crate to
handle the computations.
Features
- Ray vs. Rect Collision Detection: Detect collisions between a ray and a rectangle, returning contact point, contact normal, and the closest time of collision.
- Swept Rectangle Collision: Check for potential collisions as one rectangle moves towards another. Either using a delta 2D vector, or a scalar sweep in horizontal or vertical direction.
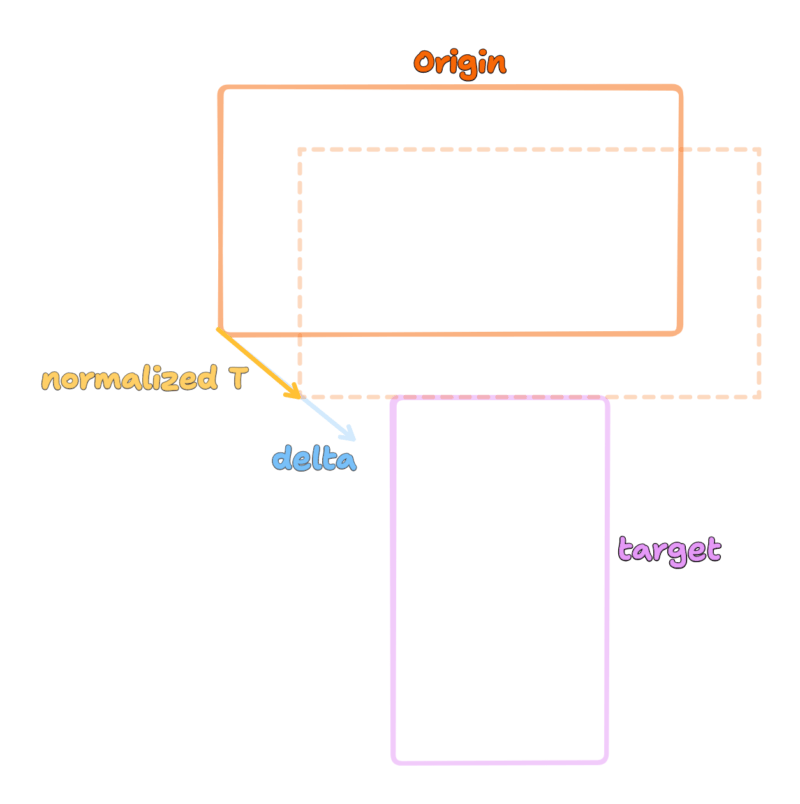
- Fixed-Point Precision: Uses fixed-point numbers (
Fp
) from thefixed32
crate for precise calculations without floating-point errors.
Usage
To use this crate in your project, add it to your Cargo.toml
:
[dependencies]
impact_rs = "0.0.17"
Example
use fixed32::Fp;
use fixed32_math::{Rect, Vector};
use impact_rs::prelude::*;
fn main() {
let ray_origin = Vector::from((1, 2));
let ray_direction = Vector::from((3, 4));
let target_rect = Rect::from((5, 6, 7, 8));
let collision_result = ray_vs_rect(ray_origin, ray_direction, target_rect);
if let Some(result) = collision_result {
println!("Collision at: {:?}", result.contact_point);
println!("Normal at collision: {:?}", result.contact_normal);
println!("Closest collision time: {:?}", result.closest_time);
} else {
println!("No collision detected.");
}
}