1 unstable release
0.1.0-beta+1 | Jul 4, 2022 |
---|
#17 in #delete-file
105KB
222 lines
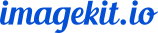
Rust API Client for ImageKit.io a file storage and image processing service
Usage
You must retrieve your Public and Private Keys from the ImageKit Developer Options.
Then create an instance of ImageKit
and initialize the client.
use imagekit::ImageKit;
use imagekit::delete::Delete;
use imagekit::upload::types::FileType;
use imagekit::upload::{Options, Upload, UploadFile};
use tokio::fs::File;
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
let mut image_kit = ImageKit::new(
"your_public_api_key",
"your_private_api_key",
"https://ik.imagekit.io/your_imagekit_id/",
);
// Upload an image from File
let file = File::open("assets/ferris.jpeg").await.unwrap();
let opts = Options::new(upload_file, "ferris");
let upload_result = imagekit.upload(opts).await.unwrap();
// Delete a file
let delete_result = imagekit.delete(upload_result.file_id).await;
}
Features
The main goal of this crate is to support the main three functionalities provided by ImageKit. URL Generation, File Upload and File Management.
The following list, provides a closer view to supported features and planned features which are not yet implemented. Feel free to contribute by opening an issue, pull request or discussion.
- URL Generation
- File Upload (File Upload API)
- From
tokio::fs::File
(Binary) - From
std::fs::File
(Binary) - From URL
- From Base64
- From
- File Management
- List Files
- Search Files
- Get File Details
- Get File Versions
- Get File Metadata
- Custom Metadata Fields
- Create
- List
- Update
- Delete
- Delete File
- Update File Details
- Tags
- Bulk Addition
- Bulk Deletion
- AI Tags
- Bulk Deletion
- Delete File Version
- Bulk Delete Files
- Copy File
- Move File
- Rename File
- Restore File Version
- Folders
- Create
- Copy
- Delete
- Move
- Bulk Job Status
- Cache
- Purge
If you notice theres missing features in this list, please open an issue or PR.
Release
In order to create a release you must push a Git tag as follows
git tag -a <version> -m <message>
Example
git tag -a v0.1.0 -m "First release"
Tags must follow semver conventions Tags must be prefixed with a lowercase
v
letter.
Then push tags as follows:
git push origin main --follow-tags
Contributing
Every contribution to this project is welcome. Feel free to open a pull request, an issue or just by starting this project.
License
As most Rust projects, this crate is licensed under both, the Apache License and the MIT License.
Dependencies
~7–19MB
~261K SLoC