191 releases (stable)
2.9.0 | Mar 10, 2025 |
---|---|
2.7.1 | Jan 27, 2025 |
2.7.0 | Dec 10, 2024 |
2.6.0 | Nov 6, 2024 |
1.8.0 | Nov 27, 2021 |
#11 in FFI
182,649 downloads per month
Used in 3 crates
(2 directly)
255KB
4.5K
SLoC
flutter_rust_bridge v2: Flutter/Dart <-> Rust binding generator, feature-rich, but seamless and simple.
What's new in V2
Tap to expand
- From 1.x to 2.0.0-dev.0:
- Rapid setup: Only a one-liner command to integrate into your project.
- Arbitrary types: Use arbitrary Rust and Dart types without manual intervention, even if they are not serializable or non-clone (previously need some manual intervention).
- Async Rust: Support asynchronous Rust (
async fn
), in addition to sync Rust / async Dart / sync Dart. - Rust call Dart: Allow Rust to call Dart functions (previously only allow Dart to call Rust).
- Support whole folders as inputs: Previously only support one single file (e.g.
api.rs
). - Use libraries/tools in Flutter/Rust: All existing libraries, Flutter debuggers, ... Nothing to stop you from using them.
- From 2.0.0-dev.0 to 2.0.0:
- Parsing third-party packages: Scan and use existing Rust packages in Dart (experimental).
- Lifetimes: Support returning types with lifetime specifiers (experimental).
- Traits: Support traits as base classes and trait objects.
- New codec: A new codec,
SSE
, which is several times faster under some workloads. - Others (>200 PRs): Auto and manual accessors, object proxies, user-defined serializers, developer experience, deadlock-free auto locking, Rust initializers, included batteries, renaming and ignoring, improving streams, more types, ...
Please visit this page for more information and update guide.
๐ What's this?
- Just write down normal Rust code (even with arbitrary types, closure,
&mut
, async, traits, etc) - And call it from Flutter, as if Rust code is normal Flutter code
- The bridge will generate all glues in between
๐ Quickstart
Create a working Flutter + Rust app and see it live, by running:
cargo install flutter_rust_bridge_codegen && flutter_rust_bridge_codegen create my_app && cd my_app && flutter run
Expand optional steps
(Optional) Edit rust/src/api/simple.rs
(e.g. Hello
-> Hi
), then see the change by:
flutter_rust_bridge_codegen generate && flutter run
For more elaborated quickstart, please visit this page.
๐ Advantages
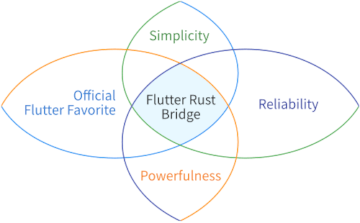
1. Officially Flutter Favorite
This package is officially Flutter Favorite, and is in the first batch of 7 packages at its rebooting. (another link)
2. Simplicity
(Tap to expand) Rapid setup, Write your code naturally, Use libraries/tools in Flutter/Rust, Battery included
- Rapid setup: Only a one-liner command to integrate into your project.
- Write your code naturally: Use your intuition and write the code you want. The bridge understands many advanced grammars (see below), allowing seamless calling Rust from Dart.
- Use libraries/tools in Flutter/Rust: All existing libraries, Flutter debuggers, ... Nothing to stop you from using them.
- Battery included: Even small things like logging and enable backtraces are configured in the starter kit.
3. Powerfulness
(Tap to expand) Arbitrary types, Async & sync, Two-way road, Auto-translatable types, Parsing third-party packages, Auto safety, Customizable & bare-metal mode, Cross-platform, ...
- Arbitrary types: Use arbitrary Rust and Dart types without manual intervention, even if they are not serializable or non-clone.
- Async & sync x Rust & Dart: Multi modes for various needs - Async Dart to avoid blocking the main thread, sync Dart for places needed (e.g. Widget.build); async Rust for IO bound tasks, thread pools for CPU-heavy computations.
- Two-way road: Not only can Dart call Rust - Rust can also call Dart.
- Auto-translatable types: Lots of types can be further translated to Dart native types, e.g. complex
enum
s andstruct
s, zero-copy big arrays, errors (Result
), andStream
s (iterator). - Parsing third-party packages: Scan and use existing Rust packages in Dart (experimental).
- Auto safety: Focus on your code, and forget memory safety, malloc/free, or undefined behavior completely.
- Customizable & bare-metal mode: Provide sensible defaults, but everything (loader, handler, ...) can be customized. You can even throw all away and only use the bare minimum calling.
- Cross-platform: Support Android, iOS, Windows, Linux, MacOS, and Web.
- Other features, e.g. support whole folders as input, pure-Dart compatible, instance and static methods, ...
4. Reliability
(Tap to expand) Solid CI, Used by many people, Easy to review, Fast, Hackable, Ask questions
- Solid CI: Valgrind & sanitizers (ASAN/MSAN/LSAN) for memory/UB-related bugs, testing per platform per mode, benchmarking, test coverage, post-release, etc, all guaranteed by CI.
- Used by many people: See here for an incomplete list.
- Easy to code-review & convince yourself: This package simply simulates how humans write boilerplate code. If you want to convince yourself (or your team) that it is safe, there is not much code to track.
- Fast: It is only a thin (though feature-rich) wrapper, benchmarked on CI, and even has multiple codecs for best performance under different workloads.
- Hackable: If (for whatever reason) you want to hack the source, there are contributor guides, code is modular, and the execution logic is intuitive.
- Ask questions: Feel free to ask questions in the issue tracker, and I usually reply within hours (if not sleeping).
Why Flutter + Rust?
Tap to expand
Firstly, super briefly introduce each component (you can find much more in a lot of blogs and posts):
- Flutter: Cross-platform, hot-reload, rapid-development, flexible UI toolkit.
- Rust: Highly efficient and performant, reliable, productive.
Typical scenarios to combine them include:
- UI framework for Rust: When you want a UI framework for your Rust system.
- Use arbitrary Rust libraries in Flutter: When the desired functionality only has a library in Rust, not Dart (Flutter).
- Need high-performance code for Flutter: Rust makes it easy and performant to write multi-thread code, algorithms, data-intensive operations, SIMD code, etc.
- ...
โจ Show me the code
Example 1
Simple Rust...
fn f(a: String, b: Vec<MyEnum>) -> MyStruct { ... }
...called from Dart, without manual intervention.
print(f(a: 'Hello', b: [MyEnum.c('Tom')]));
Example 2
Suppose we implement a word dictionary in Rust:
// โฑ Arbitrarily fancy Rust types
pub struct WordDict { .. }
// โฑ Support functions & methods
impl WordDict {
// โฑ Can call Dart back โฑ Translate errors
pub fn open(chooser: impl Fn(String) -> bool) -> Result<WordDict> { .. }
// โฑ Support async & sync Dart; property getter
#[frb(sync, getter)]
// โฑ Support T/&T/&mut T
pub fn size(&self) -> u32 { .. }
// โฑ Allow async & sync โฑ Support stream (iterator)
pub async fn search(&self, keyword: String, sink: StreamSink<String>) { .. }
}
Still seamlessly call in Dart:
final dict = await WordDict.open((situation) => true);
print(dict.size);
await for (final value in dict.search('something')) { print(value); }
There are still many features not covered here, such as parsing third party packages, lifetimes, traits, auto accessors, proxies, etc.
๐ก Documentation
Check out the documentation for quickstart, full guides and more.
๐ P.S. Achieve ~60 FPS, no matter how janky the Flutter app was due to build/layout
Here is my other open-source library :) https://github.com/fzyzcjy/flutter_smooth.
โจ Acknowledgments and contributors
Firstly, I want to sincerely thank Dart, Flutter and Rust (alphabetical order). Dart provides a solid foundation for productive UI development, Flutter enables developers to make cross-platform apps with ease, and Rust empowers everyone to build reliable and efficient software. Without the languages and frameworks, this bridge connects absolutely nothing. Besides, I also want to express my thanks for conferring the official Flutter Favorite honor to the package. In addition, I also want to say thanks to the Dart, Flutter and Rust team members as well as community members, who have helped me during the development of flutter_rust_bridge by valuable discussions, insights, and actions.
Secondly, thanks goes to these wonderful contributors (emoji key following all-contributors specification):
More specifically, thanks for all these contributions:
- Desdaemon: Support not only simple enums but also enums with fields which gets translated to native enum or sealed freezed class in Dart. Support the Option type as nullable types in Dart. Support Vec of Strings type. Support tuple type. Support comments in code. Add marker attributes for future usage. Add Linux and Windows support for with-flutter example, and make CI works for that. Avoid parameter collision. Overhaul the documentation and add several chapters to demonstrate configuring a Flutter+Rust project in all five platforms. Refactor command module. Precompiled binary CI workflow. Fix bugs. Add support for the Web platform, parallel to the existing mobile/desktop platforms, via WASM and JavaScript as intermediate values. GitHub retry actions. Implement draft of opaque types. Refactor Boxed and Option. Impl list of dates and optionals. Parameter defaults. Refactor CLI. Refactor codegen errors. Refactor for performance.
- rogurotus: Add Rust opaque types, enabling arbitrary Rust structs to be used as opaque Dart objects by generating wrappers and raw Arc pointers. Also add Dart opaque types, allowing to use any Dart objects in Rust code. Extend
SyncReturn
for more types. Fix generation bug. Fix SyncReturn. Migrate to dart-sys. Update CI. Fix linters. Fix SyncReturn bug. - ngasull: Make sync mode support whatever types that classical async mode supports. Bump sdk.
- SecondFlight: Allow structs and enums to be imported from other files within the crate by creating source graph. Auto-create relevant dir. Fix
store_dart_post_cobject
error with ffigen 6.0. - lattice0: Implement hierarchy of exceptions. Support methods, such that Rust struct impls can be converted to Dart class methods. StreamSink at any argument.
- Unoqwy: Add struct mirrors, such that types in the external crates can be imported and used without redefining and copying.
- antonok-edm: Avoid converting syn types to strings before parsing to improve code and be more robust.
- sagudev: Make code generator a
lib
. Add error types. Depend oncbindgen
. Fix LLVM paths. Update deps. Fix CI errors. - surban: Support unit return type. Skip unresolvable modules. Ignore prefer_const_constructors. Non-final Dart fields.
- Roms1383: Fix build_runner calling bug. Remove global
ffigen
dependency. Improve version check. Fix enum name-variant conflicts. Support Chrono date time and UUID types. Migrate to Rust 1.64 workspace. Update and refactor CI. Update header comments. Code cleanup. - dbsxdbsx: Allow generating multiple Rust and Dart files. Fix lint. Update doc. Add logging. Loosen config. Prefix methods.
- GregoryConrad: Add doc to setup frb inside a Dart/Flutter library.
- huang12zheng: Support type aliases and nested ones. Tweak code generation. Fix rust_build_and_test on Mac. Improve CI logic and cache. Remove bridge field in model.
- trobanga: Add support for
[T;N]
structs. Addusize
support. Add a cmd argument. Separate dart tests. Fix fallible list case. Fix test compile. Fix Result + RustAutoOpaque. - MnlPhlp: Support macros and will auto expand. Allow mirror types in streams.
- SoLongAndThanksForAllThePizza: Refactor and enhance SyncReturn to support more types. Refactor post-release CI.
- hsfzxjy: Fix SyncReturn use-after-free bug.
- Cupnfish: Support arrays as function parameters. Allow multi mirror.
- alanlzhang: Add generation for Dart metadata. Enhance and fix module parser. Fix enum in struct. Fix linter. Improve hints.
- erikas-taroza: Support list of primitive enums. Make enum camelCase. Warn wrong path. Fix cargo expand.
- SiongSng: Finish implementing exception hierarchy. Fix SyncReturn bug.
- JustSimplyKyle: Also finish implementing exception hierarchy. Allow ignore function.
- Zaitam: Fix when method return struct. Partial migration to Dart 3.
- coder0xff: Discuss binding unmodified Rust. Refactor SupportedInnerType. Extra codegen tester.
- NobodyForNothing: Support impl-for partially.
- nitn3lav: Nested
struct
s withoutBox
. - mcmah309: Add cli plugin scaffold generation.
- AlienKevin: Add flutter example for macOS. Add doc for Android NDK bug. Improve migration doc.
- alexthe2: Add Option Datetime. Add empty structs. Improve doc. Add
r#
. Fix mirror enum bug. - Larpoux: Fix async generation. Update web-audio-api binding.
- patmuk: Set MSRV. Fail fast. Improve message. Support relative config. Improve multiple docs. Fix warning.
- banool: Fix pubspec parsing. Fix symbol-stripping doc.
- AlexV525: Add Dart fix. Fix folder.
- Integral-Tech: Use concat. Fix lint.
- Tienisto: Remove default enum case. Add mock init.
- satvikpendem: Add json_serializable. Update CI. Little co-work #989.
- anlumo: Fix freezed + methods. Non-clone RustOpaque. Update syn. Support custom hasher.
- temeddix: Fix broken CI. Custom num workers. Fix MacOS doc steps. Update doc. Make zero-copy defaultable.
- NightFeather0615: Fix Vec bool.
- fmeef: Add cargo feature flag.
- alexlapa: Fix DartOpaque.
- xuwaters: Support edition 2024.
- SirKrlos: Fix fvm calls.
- OfficialBoyfriend: Fix error display.
- jippeholwerda: Fix raw identifier.
- iazel: Fix lint.
- wxitcode: Add org option. Support MacOS log. Fix a typo.
- atezet: Upgrade dependencies. Follow rustfmt.
- evdokimovs: Change dependencies.
- kyrias: Use portable atomic.
- Markus43: Fix folder removal.
- Krysl: Add preamble.
- Vollbrecht: Warn absolute path.
- w-ensink: Improve doc. Fix CI. Refactor. Add tests.
- smw-wagnerma: Improve Windows encoding handling.
- powpingdone: Document JNI init and libc++_static linking.
- debanjanbasu: Document alternative NDK init.
- valeth: Rename callFfi's port.
- sccheruku: Prevent double-generating utility.
- upsuper: Refactor delegate-attr.
- jsonmona: Add import.
- MateusHBR: Add pub get.
- codercengiz: Fix mirroring bug.
- aran: Fix map + mirror. Fix pubspec. Upgrde ffigen. Replace to js_interop. Bump version. Fix typo.
- Michael-F-Bryan: Detect broken bindings.
- phlip9: Fix no-serde compilation.
- SilverMira: Fix StreamSink.
- h3x4d3c1m4l: Fix when outside folder.
- HalidOdat: Improve config method. Hint build.rs.
- Berrysoft: Fix missing symbols.
- bus710: Add a case in troubleshooting.
- Demezy: Mention troubleshooting.
- gutenfries: Bump proc-macros.
- anstadnik: Check keywords.
- syndim: Add a bracket to box.
- vhdirk: Support dashed crate.
- akkumar: External traits doc.
- rhian-cs: Add Cargo workspace doc.
- TENX-S: Improve doc. Reproduce a bug.
- CJBuchel: Remove throw.
- polypixeldev: Improve doc.
- CicadaCinema: Bump version. Improve doc.
- CosmicHorrorDev: Change deps.
- akashgurava: Partial fix.
- w1th0utnam3: Improve message.
- vincent-herlemont: Loosen version.
- canxin121: Fix permission.
- pixelshot91: Update cargokit. Fix doc link.
- TrackerSB: Bump allo-isolate.
- bubnov: Improve doc.
- Dampfwalze: Improve doc.
- samuel-cavalcanti: Improve doc.
- zaynetro: Improve doc.
- raphaelrobert: Remove oudated doc.
- DMouayad: Improve doc.
- elliotsayes: Improve doc.
- tmpfs: Improve doc.
- thomas725: Improve doc.
- juzi5201314: Improve doc.
- Voklen: Improve doc.
- svenstaro: Improve doc.
- utilForever: Fix typos.
- not-holar: Fix typos.
- Stonks3141: Fix doc credit.
- mattiasgronlund: Bump version.
- adsouza: Fix doc grammar.
- vimaxwell: Fix doc link.
- lker-dev: Fix doc link.
- jaiakash: Fix doc link.
- feber: Fix doc link.
- adam-huganir: Fix a typo.
- ernstvanderlinden: Fix a typo.
- damywise: Fix a typo.
- rustui: Fix a typo.
- escwxyz: Fix a typo.
- eltociear: Fix a typo.
- thesimplekid: Fix a typo.
Dependencies
~2โ11MB
~126K SLoC