7 releases (breaking)
0.6.0 | Aug 25, 2023 |
---|---|
0.5.0 | Aug 24, 2023 |
0.4.0 | Aug 24, 2023 |
0.3.0 | Aug 21, 2023 |
0.1.1 | Aug 17, 2023 |
#7 in #pandas
56 downloads per month
33KB
549 lines
Combee
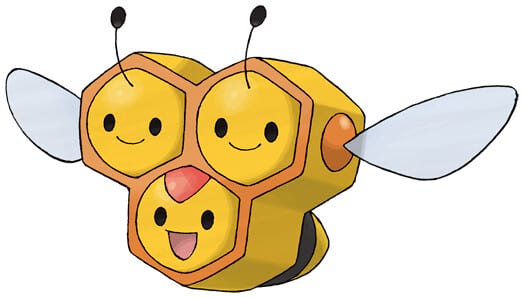
Combee is a strong typed data analysis library written in pure Rust inspired by pandas (python).
Installation
Run in a Rust project directory:
cargo add combee
Examples
-
Check the notebook using evcxr_jupyter on notebooks/analysis.ipynb to an example of analysis of dataset.
-
Below an example of loading a CSV file, filtering the dataset, and applying a function to each row:
(dataset.csv)
name,age
Daniel,26
Sergio,30
Leticia,22
(main.rs)
use serde::{Serialize, Deserialize};
use combee::{read_csv, dataframe::DataFrame};
#[derive(Clone, Deserialize, Serialize)]
struct Data {
name: String,
age: u32
}
let df = read_csv::<Data>(String::from("../tests/fixtures/basic.csv")).unwrap();
let df_filtered: DataFrame<Data> = df.filter(|row| row.age < 27);
let df_message: DataFrame<String> = df_filtered.apply(|row| format!("Hello {} with {} years!", row.name, row.age));
let messages = df_message.take(2);
println!("{}", messages[0]);
println!("{}", messages[1]);
- An example of groupby with aggregation
(main.rs)
use serde::{Serialize, Deserialize};
use combee::{read_csv, functions::{mean, sum, count, all}};
#[derive(Clone, Deserialize, Serialize)]
struct Data {
name: String,
age: u32
}
fn main() {
let df = read_csv::<Data>(String::from("dataset.csv")).unwrap();
let stats = df.groupby(all).agg(|_, g|
(count(g), mean(g, |x| x.age), sum(g, |x| x.age))
).head(1);
println("{:?}", stats);
}
Acknowledgments
Daniel Santana: Made with Love 💗.
ali5h: Code to deserialize parquet row link.
Dependencies
~28MB
~671K SLoC