11 releases (4 breaking)
0.5.1 | Aug 12, 2023 |
---|---|
0.5.0 | Aug 9, 2023 |
0.4.0 | Jul 30, 2023 |
0.3.1 | Jul 15, 2023 |
0.1.0 | Dec 26, 2022 |
#73 in Testing
Used in debctl
355KB
7K
SLoC
xpct
xpct is an extensible test assertion library for Rust. It's designed to be ergonomic, batteries-included, and test framework agnostic.
Want to get started? Check out the tutorial.
About
xpct is extensible. In addition to allowing you to write custom matchers, it separates the logic of matchers from how they format their output, meaning you can:
- Hook into existing formatters to write custom matchers with pretty output without having to worry about formatting.
- Customize the formatting of existing matchers without having to reimplement their logic.
This crate aims to provide many useful matchers out of the box. Check out the full list of provided matchers.
Docs
Examples
A simple equality assertion, like assert_eq
:
use xpct::{expect, equal};
expect!("disco").to(equal("Disco"));
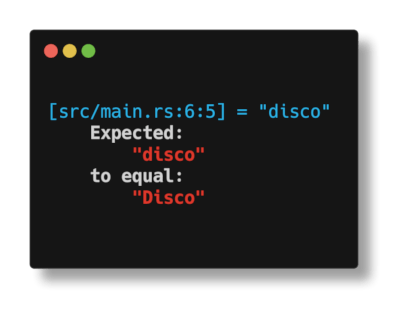
Unwrapping a Some
value to make an assertion on the wrapped value:
use xpct::{be_gt, be_some, expect};
expect!(Some(41))
.to(be_some())
.to(be_gt(57));
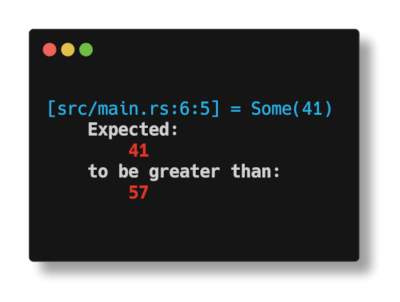
Making assertions about individual fields of a struct:
use xpct::{be_empty, be_in, be_true, expect, fields, have_prefix, match_fields, not, why};
struct Player {
id: String,
name: String,
level: u32,
is_superstar: bool,
}
let player = Player {
id: String::from("REV12-62-05-JAM41"),
name: String::from(""),
level: 21,
is_superstar: false,
};
expect!(player).to(match_fields(fields!(Player {
id: have_prefix("REV"),
name: not(be_empty()),
level: be_in(1..=20),
is_superstar: why(be_true(), "only superstars allowed"),
})));
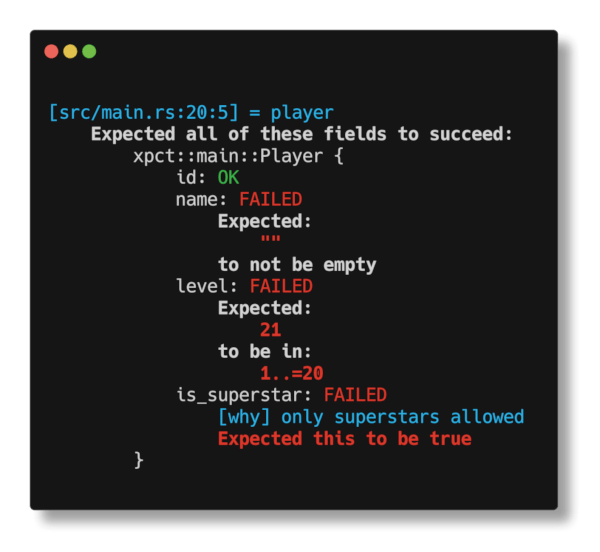
Making assertions about elements in a collection:
use xpct::{be_in, contain_substr, equal, expect, have_len, match_elements};
let items = vec!["apple", "pear", "banana"];
expect!(items)
.to(have_len(3))
.to(match_elements([
contain_substr("ana"),
equal("pear"),
be_in(["mango", "orange"]),
]));
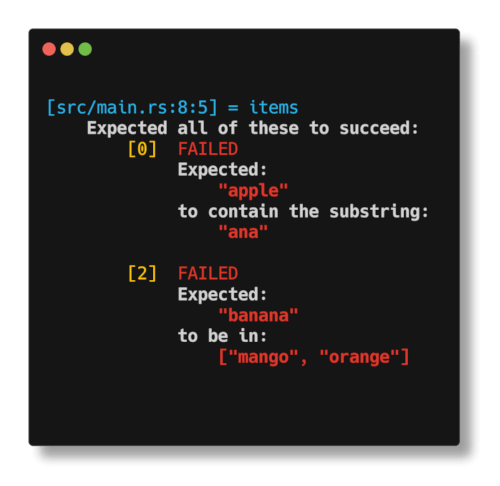
Showing rich diffs of data structures:
use xpct::{eq_diff, expect};
expect!(["apple", "banana"]).to(eq_diff(["banana", "orage"]));
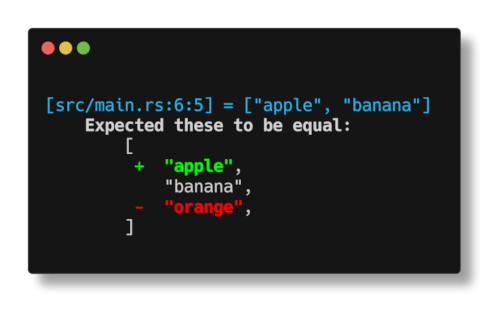
MSRV Policy
The last two stable Rust releases are supported. Older releases may be supported as well.
The MSRV will only be increased when necessary—not every time there is a new Rust release. An increase in the MSRV will be accompanied by a minor semver bump if >=1.0.0 or a patch semver bump if <1.0.0.
Semver Policy
Prior to version 1.0.0, breaking changes will be accompanied by a minor version bump, and new features and bug fixes will be accompanied by a patch version bump.
Dependencies
~0.2–10MB
~73K SLoC