2 releases
0.0.4 | Jan 23, 2024 |
---|---|
0.0.3 | Aug 9, 2023 |
#41 in #cross-platform-ui
40 downloads per month
Used in nuit
3KB
Nuit
A declarative, cross-platform UI library for Rust that uses native controls.
Nuit's API takes inspiration from SwiftUI, Xilem, React and a number of other frameworks, while itself using SwiftUI under the hood on macOS.
[!NOTE] Nuit currently requires a nightly Rust toolchain as it uses a number of cutting edge/unstable compiler features, including
- impl Trait in type aliases
- associated type defaults
- never type
!
- macro metavariable expressions
let
chainsWith
rustup
this can be configured conveniently on a per-directory basisrustup override set nightly
or, as in this repository, automatically with arust-toolchain.toml
.
Example
use nuit::{Text, VStack, View, Bind, Button, State};
#[derive(Bind)]
struct CounterView {
count: State<i32>,
}
impl CounterView {
fn new() -> Self {
Self { count: State::new(0) }
}
}
impl View for CounterView {
type Body = impl View;
fn body(&self) -> Self::Body {
let count = self.count.clone();
VStack::new((
Text::new(format!("Count: {}", count.get())),
Button::new(Text::new("Increment"), move || {
count.set(count.get() + 1);
})
))
}
}
fn main() {
nuit::run_app(CounterView::new());
}
Running this example, e.g. with cargo run --example counter
, launches:
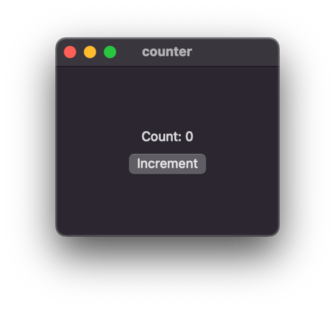
Dependencies
~295–740KB
~18K SLoC