5 unstable releases
Uses old Rust 2015
0.3.0 | Nov 25, 2017 |
---|---|
0.2.2 | Nov 12, 2017 |
0.2.1 | Nov 12, 2017 |
0.2.0 | Nov 12, 2017 |
0.1.0 | Oct 11, 2017 |
#818 in Testing
14KB
267 lines
morq

Write unit tests like humans
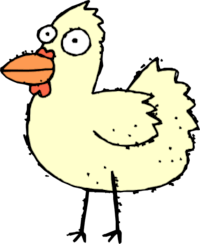
Install
cargo install morq
Morq Crate: https://crates.io/crates/morq
Grammar
Since we are using a macro here, you need to wrap the following rules in morq!
macro.
Example:
morq!(
expect(3).to.be.an(i32);
);
Chains
You use following chains to make the assertions more user friendly and readable.
- to
- be
- have
Equal
expect(30).to.be.equal(10 * 3);
expect(3).to.be.equal(1 + 2);
Close
To compare two given float
values
expect(3f32).to.be.close(3.0001f32);
expect(3f32).to.be.close_to(3.0001f32);
Not
Negates the chain.
expect(30).to.not.be.equal(10);
expect(3).to.not.be.equal(1);
expect(vec![1, 2, 3]).to.not.be.a(Vec<char>);
A / An
To check the data type.
expect(30).to.be.an(i32);
expect("hola".to_string()).to.not.be.a(f32);
expect(vec![1, 2, 3]).to.be.a(Vec<i32>);
Empty
To check and see if the iterator is empty or not
expect(vec![1, 2, 3]).to.not.be.empty();
expect(0..2).to.not.be.empty();
LengthOf
To check the count of elements in an iterator
expect(vec![1, 2, 3]).to.not.have.length_of(1usize);
expect(0..3).to.have.length_of(3usize);
Contain
Given iterator must contain the element
expect(vec![1, 2, 3]).to.contain(2);
expect(vec![false, false]).to.not.contain(true);
Ok / Err
To check a Result enum
let res: Result<String, String> = Ok(format!("boo"));
morq!(
expect(res).to.be.ok();
);
let res: Result<String, String> = Err(format!("boo"));
morq!(
expect(res).to.be.err();
);
Of course, you can combine it with not
:
let res: Result<String, String> = Err(format!("boo"));
morq!(
expect(res).to.not.be.ok();
);
Roadmap
-
Adding more chain rules
-
Adding more assert (terminal)
-
Ability to add two or more asserts in one chain:
expect("hello").to.be.equal("hello").and.not.be.a(i32);
FAQ
morq?
Means chicken in Farsi. Like a lazy chicken, you know.
Artwork: clipart-library.com
Author
Afshin Mehrabani
License
MIT
Inspired by http://chaijs.com and https://github.com/carllerche/hamcrest-rust
Dependencies
~240KB