16 releases
0.6.0 | Jun 6, 2022 |
---|---|
0.5.1 | Sep 28, 2021 |
0.5.0-beta | Jan 31, 2021 |
0.4.0 | Jan 22, 2020 |
0.3.0-async-preview-1 | Jul 30, 2019 |
#1717 in Web programming
58 downloads per month
120KB
1.5K
SLoC
lta-rs
🚍 Singapore LTA Datamall async first Rust client. lta-rs is used to interact with lta-datamall
lta-rs in action
Cargo.toml setup
[dependencies]
# extra features available: blocking
lta = { version = "0.6.0" }
API key setup
You can get your API key from here
use lta::{LTAResult, LTAClient, Client, Traffic, TrafficRequests};
#[tokio::main]
async fn main() -> LTAResult<()> {
let api_key = std::env::var("API_KEY").expect("API_KEY not found!");
let client = LTAClient::with_api_key(api_key)?;
let erp_rates = Traffic::get_erp_rates(&client, None).await?;
println!("{:?}", erp_rates);
Ok(())
}
Examples
Getting bus timings
use lta::{LTAResult, LTAClient, Client, Bus, BusRequests};
fn get_bus_arrival() -> LTAResult<()> {
let api_key = std::env::var("API_KEY").expect("API_KEY not found!");
let client = LTAClient::with_api_key(api_key);
let arrivals = Bus::get_arrival(&client, 83139, None)?;
println!("{:?}", arrivals);
Ok(())
}
Getting other data
// All the APIs in this library are designed to be used like this
// `lta::RequestType::get_something`
// All of them return lta::utils::LTAResult<T>
// The example below is Bus::get_bus_services()
// and Traffic::get_erp_rates()
// Do note that the API calling convention is similar across all the APIs except for
// bus::get_arrival
// Most of the APIs returns only 500 record
// If you want to get records 501 - 1000 take a look at get_erp() example
use lta::{LTAResult, LTAClient, Client, Bus, Traffic, BusRequests, TrafficRequests};
async fn bus_services() -> LTAResult<()> {
let api_key = std::env::var("API_KEY").expect("API_KEY not found!");
let client = LTAClient::with_api_key(api_key)?;
let bus_services= Bus::get_bus_services(&client, None)?;
println!("{:?}", bus_services);
Ok(())
}
async fn get_erp() -> LTAResult<()> {
let api_key = std::env::var("API_KEY").expect("API_KEY not found!");
let client = LTAClient::with_api_key(api_key)?;
let erp_rates = Traffic::get_erp_rates(&client, 500)?;
println!("{:?}", erp_rates);
Ok(())
}
Custom Client
There are some instances where you might need to customise the reqwest client due to certain limitations.
use lta::r#async::client::LTAClient;
use lta::reqwest::ClientBuilder;
use std::time::Duration;
use lta::Client;
fn my_custom_client() -> LTAClient {
let client = ClientBuilder::new()
.no_gzip()
.connect_timeout(Duration::new(420, 0))
.build()
.unwrap();
LTAClient::new("API_KEY", client)
}
General advice
- Reuse
LTAClient
as it holds a connection pool internally - Reduce the number of times you call the API, take a look at
Update Freq
in the documentation and prevent yourself from getting blacklisted. Use a caching mechanism.
Getting help
- You can get help via GitHub issues. I will try my best to respond to your queries 😄
Changelog
Changelog can be found here
Requirements
- Rust compiler 1.56
Frequently Asked Questions
-
Is this library being actively developed?
- Project is currently in maintenance mode. Won't really have any new features. Just bug fixes, minor upgrades etc.
-
What are the APIs available?
- All of the APIs are implemented. Take a look at the official LTA docs.
-
Where do I get the official docs from lta?
- You can get them here
License
lta-rs is licensed under MIT license (LICENSE-MIT or http://opensource.org/licenses/MIT)
Donations
For Singapore based users, you can donate using paylah!
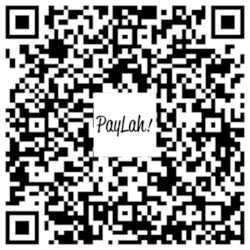
Dependencies
~7–22MB
~328K SLoC