5 releases (3 breaking)
0.4.1 | Sep 26, 2022 |
---|---|
0.4.0 | Sep 26, 2022 |
0.3.1 | May 29, 2022 |
0.2.0 | Apr 23, 2022 |
0.1.0 | Apr 21, 2022 |
#1919 in Database interfaces
110KB
3.5K
SLoC
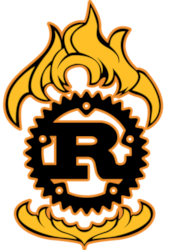
ironworks
Modular FFXIV data toolkit written in rust.
ironworks is pre-1.0, and as such its API should be considered unstable. Breaking API changes will be published on new minor versions.
To minimise unused code & dependencies, ironworks is split into a number of discrete features. No features are enabled by default - pick the ones you want to use!
Feature | Description |
---|---|
excel |
Read data from Excel databases. |
ffxiv |
Bindings for using ironworks with FFXIV. |
sqpack |
Navigate and extract files from the SqPack package format. |
Additionally, file type readers are opt-in. The feature modules above will automatically enable the file types they need, however if you need additional file types for bespoke purposes, they can be enabled manually. File type features are named by the file's extension, i.e. exl
for .exl
files.
Getting started
[dependencies]
ironworks = {version = "0.4.1", features = ["excel", "ffxiv", "sqpack"]}
use ironworks::{excel::Excel, ffxiv, file::exl, sqpack::SqPack, Error, Ironworks};
fn main() -> Result<(), Error> {
// Build the core ironworks instance. Additional resources can be registered
// for more complicated file layouts.
let ironworks = Ironworks::new()
.with_resource(SqPack::new(ffxiv::FsResource::search().unwrap()));
// Read out files as raw bytes or structured data.
let bytes = ironworks.file::<Vec<u8>>("exd/root.exl")?;
let list = ironworks.file::<exl::ExcelList>("exd/root.exl")?;
// Read fields out of excel.
let excel = Excel::with()
.language(ffxiv::Language::English)
.build(&ironworks, ffxiv::Mapper::new());
let field = excel.sheet("Item")?.row(37362)?.field(0)?;
Ok(())
}
Using generated sheets from Excel
In addition to reading individual fields as shown above, it's possible to read entire rows at a time into a struct. To faciliate this, generated sheet definitions are available as a git dependency.
Warning: The data used to generate these structs does not provide any stability guarantees whatsoever. As such, any update to sheet structs should be considered as a semver-major update.
[dependencies]
# ...
ironworks_sheets = {git = "https://github.com/ackwell/ironworks", branch = "sheets/saint-coinach"}
// ...
use ironworks_sheets::{for_type, sheet};
fn main() -> Result<(), Error> {
// ...
let field = excel.sheet(for_type::<sheet::Item>())?.row(37362)?.singular;
// ...
}
Dependencies
~2MB
~49K SLoC