image-conv
Rust library for image convolution.
Example usage
- Apply horizontal Sobel filter:
use image_conv::conv;
use image_conv::{Filter, PaddingType};
use photon_rs::native::{open_image, save_image};
fn main() {
// Open an image
let img = open_image("img.jpg").expect("No such file found");
// Create a filter
let sobel_x: Vec<f32> = vec![1.0, 0.0, -1.0, 2.0, 0.0, -2.0, 1.0, 0.0, -1.0];
let filter = Filter::from(sobel_x, 3, 3);
// Apply convolution
let img_conv = conv::convolution(&img, filter, 1, PaddingType::UNIFORM(1));
save_image(img_conv, "img_conv.jpg");
}
Some example ouputs
Original |
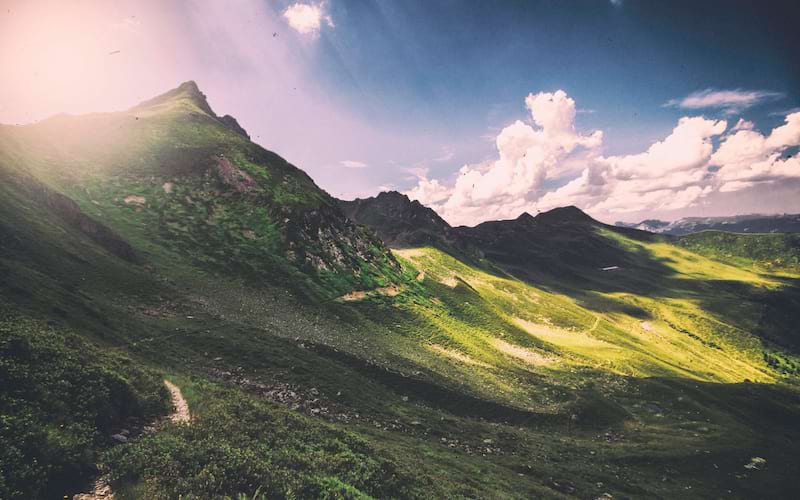 |
Sobel-X |
Sobel-Y |
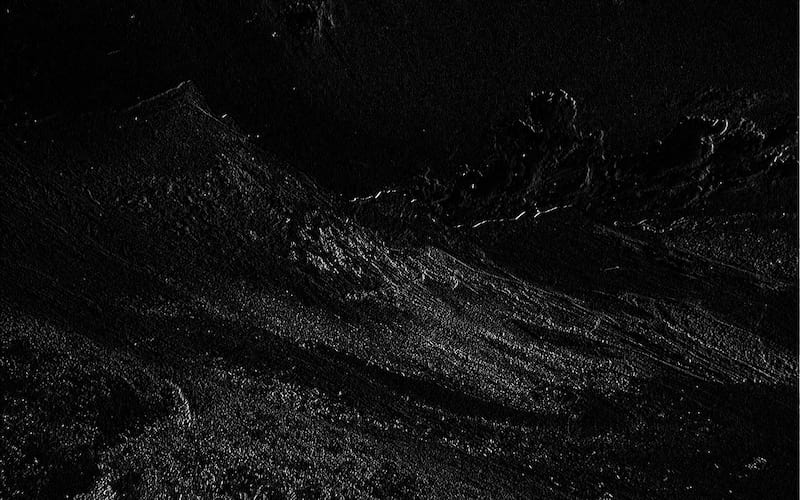 |
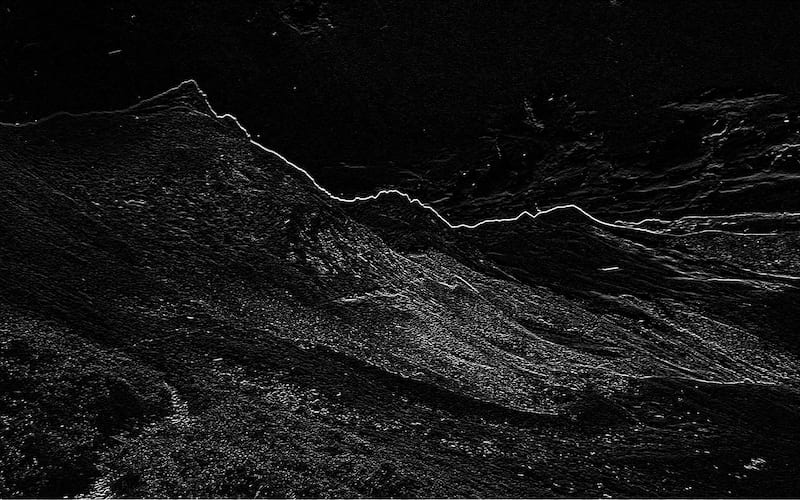 |
Scharr-X |
Scharr-Y |
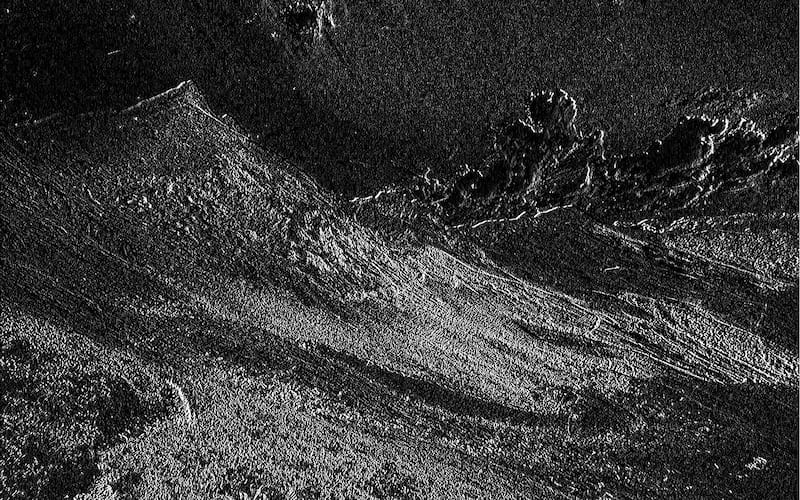 |
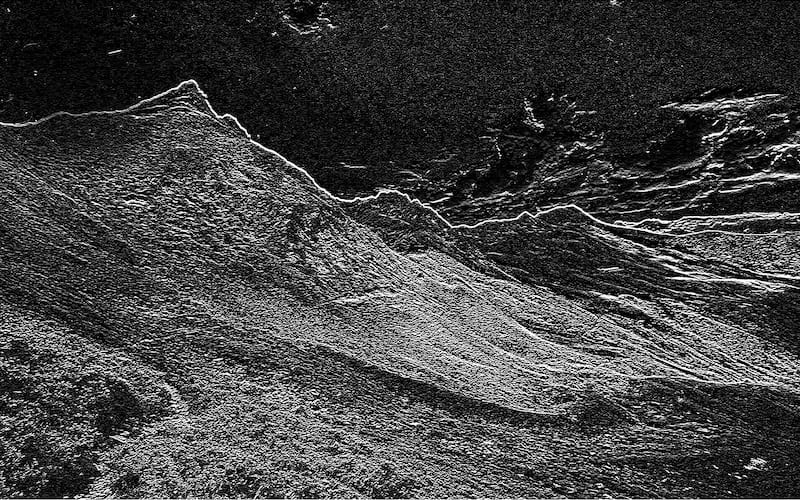 |
Laplacian |
Median |
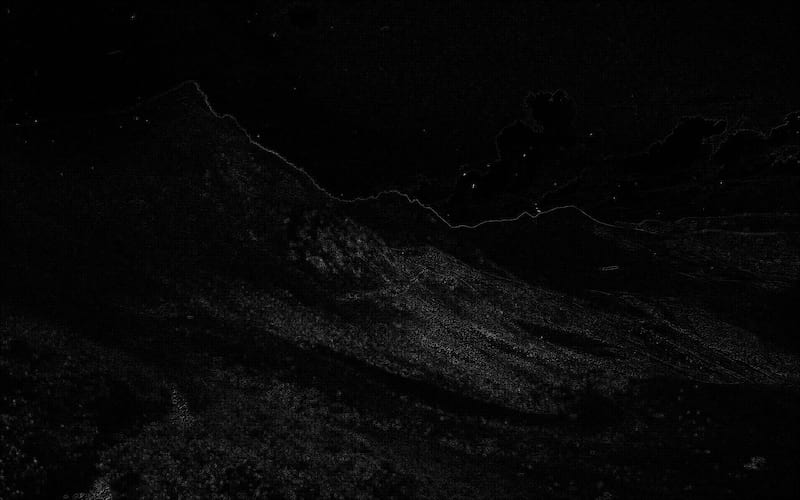 |
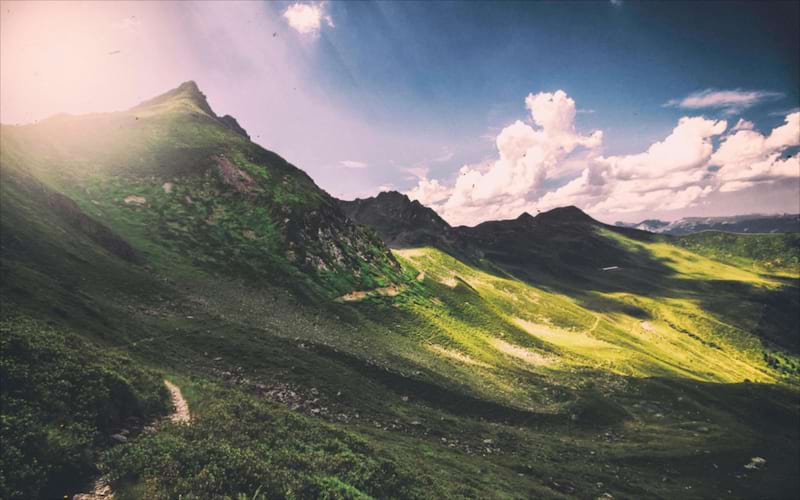 |
Gaussian |
Denoise |
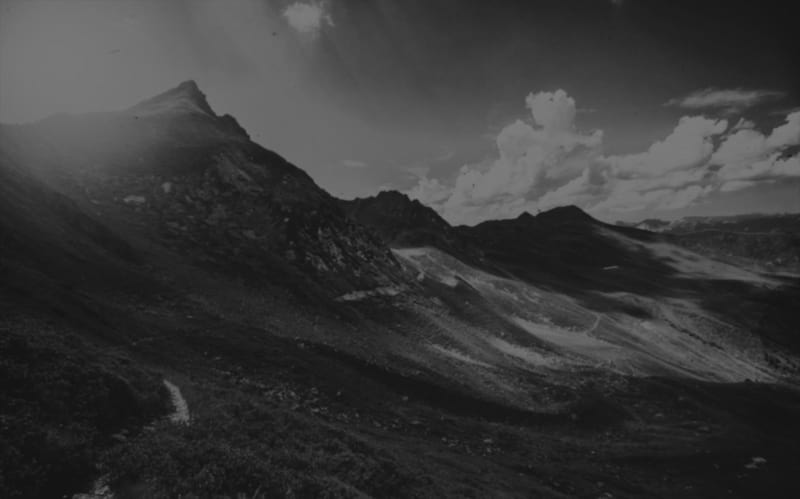 |
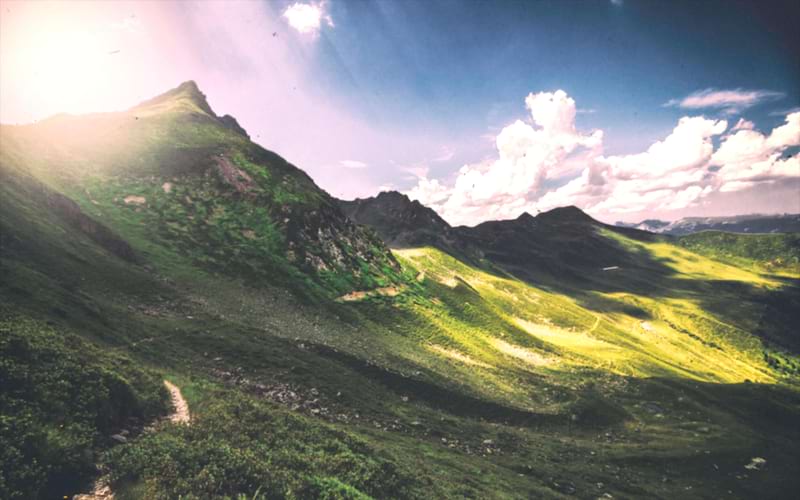 |