2 unstable releases
0.2.0 | May 20, 2021 |
---|---|
0.1.0 | Aug 3, 2020 |
#398 in Configuration
Used in elasticli
33KB
527 lines
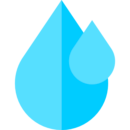
Hydroconf is a configuration management library for Rust, based on config-rs and heavily inspired by Python's dynaconf.
Features
- Inspired by the 12-factor configuration principles
- Effective separation of sensitive information (secrets)
- Layered system for multi environments (e.g. development, staging, production, etc.)
- Sane defaults, with a 1-line configuration loading
- Read from JSON, TOML, YAML, HJSON, INI files
The config-rs library is a great building block, but it does not provide a default mechanism to load configuration and merge secrets, while keeping the different environments separated. Hydroconf fills this gap.
Quickstart
Suppose you have the following file structure:
├── config
│ ├── .secrets.toml
│ └── settings.toml
└── your-executable
settings.toml
:
[default]
pg.port = 5432
pg.host = 'localhost'
[production]
pg.host = 'db-0'
.secrets.toml
:
[default]
pg.password = 'a password'
[production]
pg.password = 'a strong password'
Then, in your executable source (make sure to add serde = { version = "1.0", features = ["derive"] }
to your dependencies):
use serde::Deserialize;
use hydroconf::Hydroconf;
#[derive(Debug, Deserialize)]
struct Config {
pg: PostgresConfig,
}
#[derive(Debug, Deserialize)]
struct PostgresConfig {
host: String,
port: u16,
password: String,
}
fn main() {
let conf: Config = match Hydroconf::default().hydrate() {
Ok(c) => c,
Err(e) => {
println!("could not read configuration: {:#?}", e);
std::process::exit(1);
}
};
println!("{:#?}", conf);
}
If you compile and execute the program (making sure the executable is in the
same directory where the config
directory is), you will see the following:
$ ./your-executable
Config {
pg: PostgresConfig {
host: "localhost",
port: 5432,
password: "a password"
}
}
Hydroconf will select the settings in the [default]
table by default. If you
set ENV_FOR_HYDRO
to production
, Hydroconf will overwrite them with the
production ones:
$ ENV_FOR_HYDRO=production ./your-executable
Config {
pg: PostgresConfig {
host: "db-0",
port: 5432,
password: "a strong password"
}
}
Settings can always be overridden with environment variables:
$ HYDRO_PG__PASSWORD="an even stronger password" ./your-executable
Config {
pg: PostgresConfig {
host: "localhost",
port: 5432,
password: "an even stronger password"
}
}
The description of all Hydroconf configuration options and how the program configuration is loaded can be found in the documentation.
Dependencies
~4MB
~83K SLoC