3 releases
new 0.1.3 | May 15, 2024 |
---|---|
0.1.2 | Apr 16, 2024 |
0.1.1 | Apr 16, 2024 |
#236 in Algorithms
231 downloads per month
200KB
3.5K
SLoC
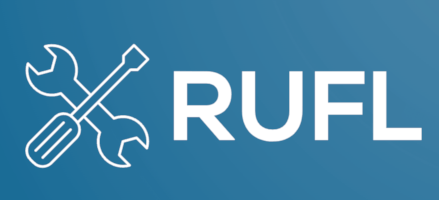
Rufl is an util function library for rust. It provides a series of useful functions to make your rust development easier.
Rufl is short for "rust util function library".
简体中文
Features
- Powerful: supports commonly used development features, string, collection, random, file... etc.
- Pure: keep external dependencies to a minimum.
- Simple: well structure, test for every function.
Installation
- cargo add rufl
[dependencies]
rufl = "0.1.3"
Example
Here takes the string function add_commas
(Add comma to a number value by every 3 numbers from right. Ahead by prefix symbol.) as an example, and the rufl::string
mod needs to be used.
Code:
use rufl::string;
fn main() {
let money_amount: String = string::add_commas("1234567", "$");
println!("current money is {}", money_amount); // current money is $1,234,567
}
Documentation
Index
1. Collection mod contains several utility functions to manipulate collection data type. index
use rufl::collection;
-
all_match: Returns true if all elements of the collection pass the predicate function check. [doc]
-
chunk: Returns a vector of elements split into groups the length of size. [doc]
-
count: Returns the number of occurrences of the given element in the collection. [doc]
-
count_by: Iterates over elements of collection with predicate function, returns the number of all matched elements. [doc]
-
difference: Creates a vector of values not included in the given collections using equality comparisons. [doc]
-
difference_by: Like difference except that it accepts iteratee which is invoked for each element of collection and values to generate the criterion by which they’re compared. [doc]
-
difference_with: Like difference except that it accepts comparator which is invoked to compare elements of collection to values. [doc]
-
fill: Fills elements of vector with initial value. [doc]
-
filter: Iterates over elements of collection, returning a collection of all elements pass the predicate function. [doc]
-
filter_map: Returns a collection which apply both filtering and mapping to the given collection. [doc]
-
find: Iterates over elements of collection, returning the first one and its index that pass predicate function. [doc]
-
find_last: Iterates over elements of collection, returning the last one and its index that pass predicate function. [doc]
-
index_of: Returns the index at which the first occurrence of a element is found in the collection. [doc]
-
insert_at: Inserts an element at position index within the vector. [doc]
-
intersection: Creates a vector of unique elements that included by the all collections. [doc]
-
is_ascending_order: Checks if all elements are in ascending order within collection. [doc]
-
is_descending_order: Checks if all elements are in descending order within collection. [doc]
-
is_sorted: Checks if all elements are sorted(ascending or descending order) within collection. [doc]
-
last_index_of: Returns the index at which the last occurrence of a element is found in the collection. [doc]
-
map: Creates new collection of element by running each element in collection thru iteratee. [doc]
-
max: Returns the maximum value of a collection. [doc]
-
min: Returns the minimum value of a collection. [doc]
-
none_match: Returns true if there is no element of the collection pass the predicate function check. [doc]
-
partition: Partition collection elements with the evaluation of the given predicate function. [doc]
-
reduce: Reduces collection to a value which is the accumulated result of running each element in collection thru iteratee [doc]
-
reduce_right: Reduce right like reduce except that it iterates over elements of collection from right to left. [doc]
-
remove_all: Remove all specific elements within the vector. [doc]
-
replace_all: Replace all old items with new items within the vector. [doc]
-
replace_n: Replace the first count n old elements with new elements in the vector. [doc]
-
shuffle: Returns a vector of shuffled values. [doc]
-
some_match: Returns true if any element of the collection pass the predicate function check. [doc]
-
union: Creates a vector of unique elements between all collections. [doc]
-
union_by: Creates a vector of unique elements between two collections. it accepts iteratee which is invoked for each element of each collection to generate the criterion by which uniqueness is computed. [doc]
-
unique: Remove duplicate elements in collection(array, vector), use PartialEq equality comparisons. [doc]
-
unique_by: Calls a provided custom comparator with element of collection, returns a vector of unique element. [doc]
2. Eventbus implements a simple pub/sub event lib.index
use rufl::eventbus;
-
Event: An event is a struct that can hold any data type. It is then published to the event bus. Once published, the event is then passed to each subscriber when the event bus runs. [doc]
-
EventBus: The event bus is a central hub for all events. It is responsible for managing all subscribers and publishing events related to the event bus. [doc]
3. File mod contains several utility functions for handling file operation.index
use rufl::file;
-
clear: Clear file content. [doc]
-
copy_dirs: Copys all directories in src path to dst path. [doc]
-
create: Creates a file in path and returns it. [doc]
-
file_names: Returns all file names of specific directory path. [doc]
-
get_md5: Gets the md5 value of file. [doc]
-
is_symlink: Checks if file is symbol link file. [doc]
-
read_to_buffer: Reads file to buffer byte array. [doc]
-
read_to_lines: Reads file and returns lines string vector. [doc]
-
read_to_string: Reads file to string. [doc]
-
write_to: Write data to file, if file isn’t exist, create it. [doc]
4. Math mod contains several utility functions for handling mathematical calculations.index
use rufl::math;
-
abs: Returns the absolute value of number n. [doc]
-
average: Calculats the average value of number vector. [doc]
-
factorial: Calculats the factorial value of number n. [doc]
-
fib_nth: Calculates the nth value of fibonacci number sequence. [doc]
-
fib_seq: Returns fibonacci number sequence. [doc]
-
fib_sum: Calculates the sum value of fibonacci number sequence. [doc]
-
gcd: Returns greatest common divisor (GCD) of integers. [doc]
-
harmonic: Calculates harmonic value number n. [doc]
-
is_prime: Checks if number is prime or not. [doc]
-
lcm: Return least common multiple (lcm) of integers. [doc]
-
percent: Calculates percentage. [doc]
-
round: Round off n decimal places to number. [doc]
-
round_down: Round down and truncate off n decimal places to number. [doc]
-
round_up: Round up and truncate off n decimal places to number. [doc]
-
sqrt: Calculates square root of float number n. [doc]
-
sum: Calculats the sum of number vector. [doc]
-
to_angle: Converts radian value to angle value. [doc]
-
to_radian: Converts angle value to radian value. [doc]
-
truncate: Truncate number to n decimal places after decimal point. [doc]
5. Random mod contains several utility functions for generating random number or string.index
use rufl::random;
-
alpha_number: Generate random alphabetic or numberic string. [doc]
-
alphabet: Generate random alphabetic string. [doc]
-
lower: Generate random lower case English letter string. [doc]
-
numberic: Generate random numberic string. [doc]
-
symbol: Generate random string which only contains special chars (!@#$%^&*()_+-=[]{}|;’:",./<>?). [doc]
-
string: Generate random string. (all kinds chars: alphabet, number, symbol). [doc]
-
upper: Generate random upper case English letter string. [doc]
6. String mod contains several utility functions for handling string.index
use rufl::string;
-
add_commas: Add comma to a number value by every 3 numbers from right. Ahead by prefix symbol. [doc]
-
after: Returns the substring after the first occurrence of a specified substr in the source string. [doc]
-
after_last: Returns the substring after the last occurrence of a specified substr in the source string. [doc]
-
before: Returns the substring before the first occurrence of a specified substr in the source string. [doc]
-
before_last: Returns the substring before the last occurrence of a specified substr in the source string. [doc]
-
camel_case: Converts string to camel case.[doc]
-
capitalize: Converts the first character of string to upper case and the remaining to lower case.[doc]
-
count_by: Counts the characters in target string with predicate function, returns the number of all matched characters. [doc]
-
count_chars: Returns the characters count in target string. [doc]
-
count_graphemes: Returns the graphemes count in target string. [doc]
-
count_words: Returns the word count in target string. [doc]
-
cut: Cut searches for the substring ‘sep’ in the source string, and splits the source string into two parts at the first occurrence of the substring ‘sep’: before and after. [doc]
-
hide: Hides some chars in source string and replace with speicfic substring. [doc]
-
index: Searches a string and returns the index of the first occurrence of the specified searched substring. [doc]
-
index_all: Searches a string and returns all the indexs of the occurrence of the specified searched substring. [doc]
-
is_alpha: Checks if the string contains only alphabetic characters. [doc]
-
is_alphanumberic: Checks if the string contains only alphabetic or numeric characters. [doc]
-
is_digit: Checks if the string contains only digit characters.(0-9) [doc]
-
is_dns: Checks if the string is a valid domain name. [doc]
-
is_email: Checks if the string is a valid email address. [doc]
-
is_ipv4: Checks if the string is a valid ipv4 address. [doc]
-
is_ipv6: Checks if the string is a valid ipv6 address. [doc]
-
is_lowercase: Checks if the string contains only lowercase unicode characters. [doc]
-
is_numberic: Checks if the string numeric (can be parsed to number). [doc]
-
is_strong_password: Checks if the string is a strong password. [doc]
-
is_uppercase: Checks if the string contains only uppercase unicode characters. [doc]
-
is_url: Checks if the string is a valid url. [doc]
-
kebab_case: Converts string to kebab case. [doc]
-
last_index: Searches a string and returns the index of the last occurrence of the specified searched substring. [doc]
-
lower_first: Converts the first character of string to lower case. [doc]
-
pad: Pads string on the left and right sides if it’s shorter than length. Padding characters are truncated if they can’t be evenly divided by length. [doc]
-
pad_end: Pads string on the right side if it’s shorter than length. Padding str are truncated if they exceed length. [doc]
-
pad_start: Pads string on the left side if it’s shorter than length. Padding str are truncated if they exceed length. [doc]
-
pascal_case: Converts string to pascal case. [doc]
-
remove_all: Removes all the specified substring which occurrence in the source string. [doc]
-
remove_first: Removes the specified substring which first occurrence in the source string. [doc]
-
remove_last: Removes the specified substring which last occurrence in the source string. [doc]
-
removen: Removes first n matches substr in the source string. [doc]
-
snake_case: Converts string to snake case. [doc]
-
split_chars: Split the input string into a vector of characters. [doc]
-
split_graphemes: Split the target string into a vector of graphemes str. [doc]
-
split_words: Split the input string into a vector of its words (based on the position of capital letters or numbers). [doc]
-
starts_with_offset: Checks whether a string starts with the specified prefix at offset position. [doc]
-
substring: Returns the part of target string from the start index up to and excluding the end index. [doc]
-
title_case: Converts string to title case. [doc]
-
upper_first: Converts the first character of string to upper case. [doc]
How to Contribute
Contributing Guide
Dependencies
~2.9–4MB
~69K SLoC